- 30 Aug, 2023 2 commits
-
-
Taha Tesser authored
fixes [Unable to adjust the color for "Cancel" and "Ok" button in datePicker dialog](https://github.com/flutter/flutter/issues/127739) ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, theme: ThemeData( useMaterial3: true, datePickerTheme: DatePickerThemeData( cancelButtonStyle: TextButton.styleFrom( shape: const RoundedRectangleBorder( borderRadius: BorderRadius.all(Radius.circular(16)), side: BorderSide(color: Colors.red), ), backgroundColor: Colors.white, foregroundColor: Colors.red, elevation: 3, shadowColor: Colors.red, ), confirmButtonStyle: TextButton.styleFrom( shape: const RoundedRectangleBorder( borderRadius: BorderRadius.all(Radius.circular(16)), ), backgroundColor: Colors.green[700], foregroundColor: Colors.white, elevation: 3, shadowColor: Colors.green[700], ), ), ), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: DatePickerDialog( initialDate: DateTime.now(), firstDate: DateTime(2020), lastDate: DateTime(2030), ), ), ); } } ``` </details> ### Before Not possible to customize action buttons from the `DatePickerThemeData`. ### After 
-
Xilai Zhang authored
Reverts flutter/flutter#132941 context: b/298110031 The rounded rectangle borders don't appear in some of the internal golden image tests.
-
- 29 Aug, 2023 1 commit
-
-
Greg Spencer authored
-
- 28 Aug, 2023 1 commit
-
-
Greg Spencer authored
-
- 25 Aug, 2023 2 commits
-
-
Kenzie Davisson authored
Generated by running `flutter update-packages --force-upgrade`
-
Taha Tesser authored
fixes [Chip border side color not working in Material3](https://github.com/flutter/flutter/issues/132922) ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( useMaterial3: true, chipTheme: const ChipThemeData( // shape: RoundedRectangleBorder( // side: BorderSide(color: Colors.amber), // borderRadius: BorderRadius.all(Radius.circular(12)), // ), // side: BorderSide(color: Colors.red), ), ), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return const Scaffold( body: Center( child: RawChip( shape: RoundedRectangleBorder( side: BorderSide(color: Colors.amber), borderRadius: BorderRadius.all(Radius.circular(12)), ), // side: BorderSide(color: Colors.red), label: Text('Chip'), ), ), ); } } ``` </details> --- ### Before When `RawChip.shape` is provided with a `BorderSide`. ```dart body: Center( child: RawChip( shape: RoundedRectangleBorder( side: BorderSide(color: Colors.amber), borderRadius: BorderRadius.all(Radius.circular(12)), ), label: Text('Chip'), ), ), ```  When `RawChip.shape` is provided with a `BorderSide` and also `RawChip.side` is provided. The `RawChip.side` overrides the shape's side. ```dart body: Center( child: RawChip( shape: RoundedRectangleBorder( side: BorderSide(color: Colors.amber), borderRadius: BorderRadius.all(Radius.circular(12)), ), side: BorderSide(color: Colors.red), label: Text('Chip'), ), ), ```  --- ### After When `RawChip.shape` is provided with a `BorderSide`. ```dart body: Center( child: RawChip( shape: RoundedRectangleBorder( side: BorderSide(color: Colors.amber), borderRadius: BorderRadius.all(Radius.circular(12)), ), label: Text('Chip'), ), ), ```  When `RawChip.shape` is provided with a `BorderSide` and also `RawChip.side` is provided. The `RawChip.side` overrides the shape's side. ```dart body: Center( child: RawChip( shape: RoundedRectangleBorder( side: BorderSide(color: Colors.amber), borderRadius: BorderRadius.all(Radius.circular(12)), ), side: BorderSide(color: Colors.red), label: Text('Chip'), ), ), ```  ---
-
- 24 Aug, 2023 1 commit
-
-
Ian Hickson authored
-
- 22 Aug, 2023 1 commit
-
-
Taha Tesser authored
Related https://github.com/flutter/flutter/issues/131676 ## Description #### Fix default input text style for `DropdownMenu`  ### Fix default text style for `MenuAnchor`'s menu items (which `DropdownMenu` uses for menu items)  ### Default `DropdownMenu` Input text style  ### Default `DropdownMenu` menu item text style  ### Default `MenuAnchor` menu item text style  ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [DropdownMenu]s. The first dropdown menu has an outlined border. void main() => runApp(const DropdownMenuExample()); class DropdownMenuExample extends StatefulWidget { const DropdownMenuExample({super.key}); @override State<DropdownMenuExample> createState() => _DropdownMenuExampleState(); } class _DropdownMenuExampleState extends State<DropdownMenuExample> { final TextEditingController colorController = TextEditingController(); final TextEditingController iconController = TextEditingController(); ColorLabel? selectedColor; IconLabel? selectedIcon; @override Widget build(BuildContext context) { final List<DropdownMenuEntry<ColorLabel>> colorEntries = <DropdownMenuEntry<ColorLabel>>[]; for (final ColorLabel color in ColorLabel.values) { colorEntries.add( DropdownMenuEntry<ColorLabel>( value: color, label: color.label, enabled: color.label != 'Grey'), ); } final List<DropdownMenuEntry<IconLabel>> iconEntries = <DropdownMenuEntry<IconLabel>>[]; for (final IconLabel icon in IconLabel.values) { iconEntries .add(DropdownMenuEntry<IconLabel>(value: icon, label: icon.label)); } return MaterialApp( theme: ThemeData( useMaterial3: true, colorSchemeSeed: Colors.green, // textTheme: const TextTheme( // bodyLarge: TextStyle( // fontWeight: FontWeight.bold, // fontStyle: FontStyle.italic, // decoration: TextDecoration.underline, // ), // ), ), home: Scaffold( body: SafeArea( child: Column( children: <Widget>[ const Text('DropdownMenus'), Padding( padding: const EdgeInsets.symmetric(vertical: 20), child: Row( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ DropdownMenu<ColorLabel>( controller: colorController, label: const Text('Color'), dropdownMenuEntries: colorEntries, onSelected: (ColorLabel? color) { setState(() { selectedColor = color; }); }, ), const SizedBox(width: 20), DropdownMenu<IconLabel>( controller: iconController, enableFilter: true, leadingIcon: const Icon(Icons.search), label: const Text('Icon'), dropdownMenuEntries: iconEntries, inputDecorationTheme: const InputDecorationTheme( filled: true, contentPadding: EdgeInsets.symmetric(vertical: 5.0), ), onSelected: (IconLabel? icon) { setState(() { selectedIcon = icon; }); }, ), ], ), ), const Text('Plain TextFields'), Padding( padding: const EdgeInsets.symmetric(vertical: 20), child: Row( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ SizedBox( width: 150, child: TextField( controller: TextEditingController(text: 'Blue'), decoration: const InputDecoration( suffixIcon: Icon(Icons.arrow_drop_down), labelText: 'Color', border: OutlineInputBorder(), )), ), const SizedBox(width: 20), SizedBox( width: 150, child: TextField( controller: TextEditingController(text: 'Smile'), decoration: const InputDecoration( prefixIcon: Icon(Icons.search), suffixIcon: Icon(Icons.arrow_drop_down), filled: true, labelText: 'Icon', )), ), ], ), ), if (selectedColor != null && selectedIcon != null) Row( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( 'You selected a ${selectedColor?.label} ${selectedIcon?.label}'), Padding( padding: const EdgeInsets.symmetric(horizontal: 5), child: Icon( selectedIcon?.icon, color: selectedColor?.color, ), ) ], ) else const Text('Please select a color and an icon.') ], ), ), ), ); } } enum ColorLabel { blue('Blue', Colors.blue), pink('Pink', Colors.pink), green('Green', Colors.green), yellow('Yellow', Colors.yellow), grey('Grey', Colors.grey); const ColorLabel(this.label, this.color); final String label; final Color color; } enum IconLabel { smile('Smile', Icons.sentiment_satisfied_outlined), cloud( 'Cloud', Icons.cloud_outlined, ), brush('Brush', Icons.brush_outlined), heart('Heart', Icons.favorite); const IconLabel(this.label, this.icon); final String label; final IconData icon; } ``` </details>
-
- 21 Aug, 2023 1 commit
-
-
Gray Mackall authored
The `generate_gradle_lockfiles.dart` script was generating the gradle wrapper by building a flavor that didn't exist. In the time since the script was written, the `--config-only` flag was created and should be used instead. Context https://github.com/flutter/flutter/pull/132406#discussion_r1300352602
-
- 18 Aug, 2023 1 commit
-
-
Zachary Anderson authored
It looks like the logic added here: https://github.com/flutter/flutter/pull/131951/files#diff-297ee4cfe6d9bffc2fa1376918a50b8e4165ada4569575880c40811b6f749265R18 got dropped in the refactor here: https://github.com/flutter/flutter/pull/132710
-
- 17 Aug, 2023 1 commit
-
-
Greg Spencer authored
## Description This re-lands #132353 with some additional options for keeping around the staging directory, so that the recipe for publishing docs can give those options and have the staging directory left around for deploying to the website. Reverted in #132613 ## Related Issues - https://flutter-review.googlesource.com/c/recipes/+/49580
-
- 16 Aug, 2023 3 commits
-
-
Polina Cherkasova authored
-
Jonah Williams authored
Reverts flutter/flutter#132353 Educated guess that this is causing the docs failures: https://logs.chromium.org/logs/flutter/buildbucket/cr-buildbucket/8772644466113801713/+/u/Docs/Deploy_docs/Firebase_deploy/stdout
-
Taha Tesser authored
This relands https://github.com/flutter/flutter/pull/131609 --- fixes [`PopupMenuItem` adds redundant padding when using `ListItem`](https://github.com/flutter/flutter/issues/128553)
-
- 15 Aug, 2023 2 commits
-
-
Greg Spencer authored
## Description This cleans up a lot of issues with the API doc generation. Here are the main changes: - Rename `dartdoc.dart` to `create_api_docs.dart` - Move the bulk of the operations out of `dev/bots/docs.sh` into `create_api_docs.dart`. - Delete `dashing_postprocess.dart` and `java_and_objc.dart` and incorporate those operations into `create_api_docs.dart`. - Refactor the doc generation into more understandable classes - Bump the snippets tool version to 0.4.0 (the latest one) - Centralize the information gathering about the Flutter repo into the new `FlutterInformation` class. - Clean up the directory handling, and convert to using the `file` package for all file and directory paths. - Add an `--output` option to docs.sh that specifies the location of the output ZIP file containing the docs. - Defaults to placing the output in `dev/docs/api_docs.zip` (i.e. where the previous code generates the file). - Moved all document generation into a temporary folder that is removed once the documents are generated, to avoid VSCode and other IDEs trying to index the thousands of HTML and JS files in the docs output. - Updated pubspec dependencies. ## Tests - Added tests for doc generation.
-
Polina Cherkasova authored
-
- 13 Aug, 2023 1 commit
-
-
Casey Hillers authored
Revert "Fix `PopupMenuItem` & `CheckedPopupMenuItem` has redundant `ListTile` padding and update default horizontal padding for Material 3" (#132457) Reverts flutter/flutter#131609 b/295497265 - This broke Google Testing. We'll need the internal patch before landing as there's a large number of customer codebases impacted.
-
- 10 Aug, 2023 2 commits
-
-
Polina Cherkasova authored
-
Taha Tesser authored
Fix `PopupMenuItem` & `CheckedPopupMenuItem` has redundant `ListTile` padding and update default horizontal padding for Material 3 (#131609) fixes [`PopupMenuItem` adds redundant padding when using `ListItem`](https://github.com/flutter/flutter/issues/128553) ### Description - Fixed redundant `ListTile` padding when using `CheckedPopupMenuItem` or `PopupMenuItem` with the `ListTile` child for complex popup menu items as suggested in the docs. - Updated default horizontal padding for popup menu items. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [PopupMenuButton]. // This is the type used by the popup menu below. enum SampleItem { itemOne, itemTwo, itemThree } void main() => runApp(const PopupMenuApp()); class PopupMenuApp extends StatelessWidget { const PopupMenuApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData(useMaterial3: true), home: const PopupMenuExample(), ); } } class PopupMenuExample extends StatefulWidget { const PopupMenuExample({super.key}); @override State<PopupMenuExample> createState() => _PopupMenuExampleState(); } class _PopupMenuExampleState extends State<PopupMenuExample> { SampleItem? selectedMenu; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: const Text('PopupMenuButton')), body: Center( child: SizedBox( width: 150, height: 100, child: Align( alignment: Alignment.topLeft, child: PopupMenuButton<SampleItem>( initialValue: selectedMenu, // Callback that sets the selected popup menu item. onSelected: (SampleItem item) { setState(() { selectedMenu = item; }); }, itemBuilder: (BuildContext context) => <PopupMenuEntry<SampleItem>>[ const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: Text('PopupMenuItem'), ), const CheckedPopupMenuItem<SampleItem>( checked: true, value: SampleItem.itemTwo, child: Text('CheckedPopupMenuItem'), ), const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: ListTile( leading: Icon(Icons.cloud), title: Text('ListTile'), contentPadding: EdgeInsets.zero, trailing: Icon(Icons.arrow_right_rounded), ), ), ], ), ), ), ), ); } } ``` </details> ### Before  - Default horizontal padding is the same as M2 (16.0), while the specs use a smaller value (12.0) - `ListTile` nested by default in `CheckedPopupMenuItem` has redundant padding - `PopupMenuItem` using `ListTile` as a child for complex menu items contains redundant padding.  ### After - Default horizontal padding is updated for Material 3. - `PopupMenuItem` & `CheckedPopupMenuItem` override `ListTile` padding (similar to how `ExpansionTile` overrides `ListTile` text and icon color. 
-
- 09 Aug, 2023 2 commits
-
-
Zachary Anderson authored
Context: https://github.com/flutter/flutter/issues/131862 This PR injects a "realm" component to the storage base URL when the contents of the file `bin/internal/engine.realm` is non-empty. As documented in the PR, when the realm is `flutter_archives_v2`, and `bin/internal/engine.version` contains the commit hash for a commit in a `flutter/engine` PR, then the artifacts pulled by the tool will be the artifacts built by the presubmit checks for the PR. This works for everything but the following two cases: 1. Fuchsia artifacts are not uploaded to CIPD by the Fuchsia presubmit builds. 2. Web artifacts are not uploaded to gstatic by the web engine presubmit builds. For (1), the flutter/flutter presubmit `fuchsia_precache` is driven by a shell script outside of the repo. It will fail when the `engine.version` and `engine.realm` don't point to a post-submit engine commit. For (2), the flutter/flutter web presubmit tests that refer to artifacts in gstatic hang when the artifacts aren't found, so this PR skips them.
-
Zachary Anderson authored
Reverts flutter/flutter#131998 Reverting for https://github.com/flutter/flutter/issues/132222
-
- 08 Aug, 2023 3 commits
-
-
Qun Cheng authored
This is to add `textCapitalization` property for `SearchBar` and `SearchAnchor`. Fixes: #131260
-
Ian Hickson authored
-
Polina Cherkasova authored
-
- 04 Aug, 2023 1 commit
-
-
Polina Cherkasova authored
-
- 03 Aug, 2023 1 commit
-
-
Taha Tesser authored
Fix issue reference for https://github.com/flutter/flutter/issues/131247 (it was added in the bug fix PR https://github.com/flutter/flutter/pull/131253)
-
- 02 Aug, 2023 1 commit
-
-
Taha Tesser authored
fixes [Material 3 `TabBar` does not take full width when `isScrollable: true`](https://github.com/flutter/flutter/issues/117722) ### Description 1. Fixed the divider doesn't stretch to take all the available width in the scrollable tab bar in M3 2. Added `dividerHeight` property. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [TabBar]. void main() => runApp(const TabBarApp()); class TabBarApp extends StatelessWidget { const TabBarApp({super.key}); @override Widget build(BuildContext context) { return const MaterialApp( debugShowCheckedModeBanner: false, home: TabBarExample(), ); } } class TabBarExample extends StatefulWidget { const TabBarExample({super.key}); @override State<TabBarExample> createState() => _TabBarExampleState(); } class _TabBarExampleState extends State<TabBarExample> { bool rtl = false; bool customColors = false; bool removeDivider = false; Color dividerColor = Colors.amber; Color indicatorColor = Colors.red; @override Widget build(BuildContext context) { return DefaultTabController( initialIndex: 1, length: 3, child: Directionality( textDirection: rtl ? TextDirection.rtl : TextDirection.ltr, child: Scaffold( appBar: AppBar( title: const Text('TabBar Sample'), actions: <Widget>[ IconButton.filledTonal( tooltip: 'Switch direction', icon: const Icon(Icons.swap_horiz), onPressed: () { setState(() { rtl = !rtl; }); }, ), IconButton.filledTonal( tooltip: 'Use custom colors', icon: const Icon(Icons.color_lens), onPressed: () { setState(() { customColors = !customColors; }); }, ), IconButton.filledTonal( tooltip: 'Show/hide divider', icon: const Icon(Icons.remove_rounded), onPressed: () { setState(() { removeDivider = !removeDivider; }); }, ), ], ), body: Column( children: <Widget>[ const Spacer(), const Text('Scrollable - TabAlignment.start'), TabBar( isScrollable: true, tabAlignment: TabAlignment.start, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Scrollable - TabAlignment.startOffset'), TabBar( isScrollable: true, tabAlignment: TabAlignment.startOffset, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Scrollable - TabAlignment.center'), TabBar( isScrollable: true, tabAlignment: TabAlignment.center, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Spacer(), const Text('Non-scrollable - TabAlignment.fill'), TabBar( tabAlignment: TabAlignment.fill, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Non-scrollable - TabAlignment.center'), TabBar( tabAlignment: TabAlignment.center, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Spacer(), const Text('Secondary - TabAlignment.fill'), TabBar.secondary( tabAlignment: TabAlignment.fill, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Secondary - TabAlignment.center'), TabBar.secondary( tabAlignment: TabAlignment.center, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Spacer(), ], ), ), ), ); } } ``` </details> ### Before  ### After  This also contains regression test for https://github.com/flutter/flutter/pull/125974#discussion_r1239089151 ```dart // This is a regression test for https://github.com/flutter/flutter/pull/125974#discussion_r1239089151. testWidgets('Divider can be constrained', (WidgetTester tester) async { ``` 
-
- 01 Aug, 2023 1 commit
-
-
Polina Cherkasova authored
-
- 28 Jul, 2023 1 commit
-
-
Taha Tesser authored
Fix `TimePicker` defaults for `hourMinuteTextStyle` and `dayPeriodTextColor` for Material 3 (#131253) fixes [`TimePicker` color and visual issues](https://github.com/flutter/flutter/issues/127035) ## Description - fixes default text style for `TimePicker`s `hourMinuteTextStyle` and added a todo for https://github.com/flutter/flutter/issues/131247 - fixes correct default color not being accessed for `dayPeriodTextColor` - Updates tests ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, theme: ThemeData(useMaterial3: true), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Sample'), ), body: Center( child: ElevatedButton( onPressed: () { showTimePicker( context: context, orientation: Orientation.portrait, initialEntryMode: TimePickerEntryMode.input, initialTime: TimeOfDay.now(), builder: (BuildContext context, Widget? child) { return MediaQuery( data: MediaQuery.of(context) .copyWith(alwaysUse24HourFormat: true), child: child!, ); }, ); }, child: const Text('Open Time Picker'), ), ), ); } } ``` </details> ### Before 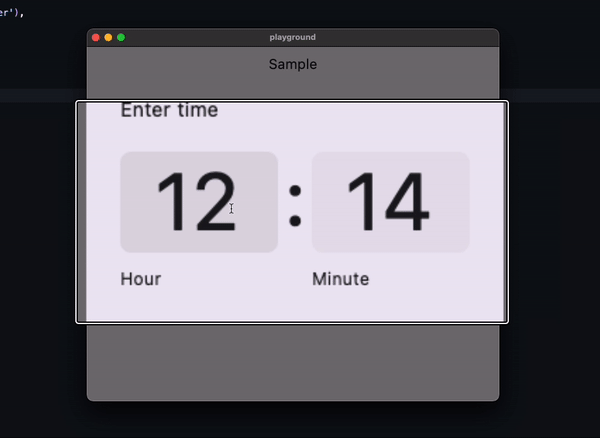 ### After 
-
- 27 Jul, 2023 1 commit
-
-
Jason Simmons authored
Dart SDK 3.2.x requires a new version of the dart_internal package.
-
- 24 Jul, 2023 1 commit
-
-
Taha Tesser authored
fixes [Material3: TimePicker clock dial use wrong spec color and its web spec has a mistake](https://github.com/flutter/flutter/issues/118657) ### Description This PR fixes the default color used for the Material 3 dial background. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { final ThemeData theme = ThemeData(useMaterial3: true); return MaterialApp( debugShowCheckedModeBanner: false, // theme: theme, theme: theme.copyWith( colorScheme: theme.colorScheme.copyWith( surfaceVariant: const Color(0xffffbf00), ), ), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Sample'), ), body: Center( child: ElevatedButton( onPressed: () { showTimePicker( context: context, initialTime: TimeOfDay.now(), ); }, child: const Text('Open Time Picker'), ), ), ); } } ``` </details> ### Default dial background color | Before | After | | --------------- | --------------- | | <img src="https://github.com/flutter/flutter/assets/48603081/59514586-60c6-489f-b024-f659a26fa1e7" /> | <img src="https://github.com/flutter/flutter/assets/48603081/75c3c360-df2b-47c8-8187-136ff6d963b6" /> | ### Custom color scheme | Before | After | | --------------- | --------------- | | <img src="https://github.com/flutter/flutter/assets/48603081/666dd2fc-7ee2-4268-9af0-923019adfccd" /> | <img src="https://github.com/flutter/flutter/assets/48603081/f32dc39e-a43f-4a63-a6e4-9df479b723ed" /> |
-
- 21 Jul, 2023 1 commit
-
-
Pierre-Louis authored
Fixes https://github.com/flutter/flutter/issues/130978 ## Pre-launch Checklist - [x] I read the [Contributor Guide] and followed the process outlined there for submitting PRs. - [x] I read the [Tree Hygiene] wiki page, which explains my responsibilities. - [x] I read and followed the [Flutter Style Guide], including [Features we expect every widget to implement]. - [x] I signed the [CLA]. - [x] I listed at least one issue that this PR fixes in the description above. - [x] I updated/added relevant documentation (doc comments with `///`). - [x] I added new tests to check the change I am making, or this PR is [test-exempt]. - [x] All existing and new tests are passing. If you need help, consider asking for advice on the #hackers-new channel on [Discord]. <!-- Links --> [Contributor Guide]: https://github.com/flutter/flutter/wiki/Tree-hygiene#overview [Tree Hygiene]: https://github.com/flutter/flutter/wiki/Tree-hygiene [test-exempt]: https://github.com/flutter/flutter/wiki/Tree-hygiene#tests [Flutter Style Guide]: https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo [Features we expect every widget to implement]: https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#features-we-expect-every-widget-to-implement [CLA]: https://cla.developers.google.com/ [flutter/tests]: https://github.com/flutter/tests [breaking change policy]: https://github.com/flutter/flutter/wiki/Tree-hygiene#handling-breaking-changes [Discord]: https://github.com/flutter/flutter/wiki/Chat
-
- 19 Jul, 2023 1 commit
-
-
Pierre-Louis authored
## Description This adds support for M3 easing and duration tokens. This PR includes these changes: * Generation of duration and easing constants, in `Durations` and `Easing`, respectively (`Curves` is already taken in the `animation` library) * Add 3 Dart fixes Once this is merged, I'll migrate packages/plugins/customers and then uncomment the deprecation notices for the 3 M2 curves, all of which have 1:1 replacements. ## Related Issues - Fixes https://github.com/flutter/flutter/issues/116525 ## Tests - Added Dart fix tests ## Pre-launch Checklist - [x] I read the [Contributor Guide] and followed the process outlined there for submitting PRs. - [x] I read the [Tree Hygiene] wiki page, which explains my responsibilities. - [x] I read and followed the [Flutter Style Guide], including [Features we expect every widget to implement]. - [x] I signed the [CLA]. - [x] I listed at least one issue that this PR fixes in the description above. - [x] I updated/added relevant documentation (doc comments with `///`). - [x] I added new tests to check the change I am making, or this PR is [test-exempt]. - [x] All existing and new tests are passing. If you need help, consider asking for advice on the #hackers-new channel on [Discord]. <!-- Links --> [Contributor Guide]: https://github.com/flutter/flutter/wiki/Tree-hygiene#overview [Tree Hygiene]: https://github.com/flutter/flutter/wiki/Tree-hygiene [test-exempt]: https://github.com/flutter/flutter/wiki/Tree-hygiene#tests [Flutter Style Guide]: https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo [Features we expect every widget to implement]: https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#features-we-expect-every-widget-to-implement [CLA]: https://cla.developers.google.com/ [flutter/tests]: https://github.com/flutter/tests [breaking change policy]: https://github.com/flutter/flutter/wiki/Tree-hygiene#handling-breaking-changes [Discord]: https://github.com/flutter/flutter/wiki/Chat
-
- 18 Jul, 2023 1 commit
-
-
flutter-pub-roller-bot authored
This PR was generated by `flutter update-packages --force-upgrade`.
-
- 17 Jul, 2023 1 commit
-
-
LongCatIsLooong authored
Deprecate `textScaleFactor` in favor of `textScaler`, in preparation for Android 14 [Non-linear font scaling to 200%](https://developer.android.com/about/versions/14/features#non-linear-font-scaling). The `TextScaler` class can be moved to `dart:ui` in the future, if we decide to use the Android platform API or AndroidX to get the scaling curve instead of hard coding the curve in the framework. I haven't put the Flutter version in the deprecation message so the analyzer checks are failing. Will do so after I finish the migration guide. **Why `TextScaler.textScaleFactor`** The author of a `TextScaler` subclass should provide a fallback `textScaleFactor`. By making `TextScaler` also contain the `textScaleFactor` information it also makes it easier to migrate: if a widget overrides `MediaQueryData.textScaler` in the tree, for unmigrated widgets in the subtree it would also have to override `MediaQueryData.textScaleFactor`, and that makes it difficult to remove `MediaQueryData.textScaleFactor` in the future. ## A full list of affected APIs in this PR Deprecated: The method/getter/setter/argument is annotated with a `@Deprecated()` annotation in this PR, and the caller should replace it with `textScaler` instead. Unless otherwise specified there will be a Flutter fix available to help with migration but it's still recommended to migrate case-by-case. **Replaced**: The method this `textScaleFactor` argument belongs to is rarely called directly by user code and is not overridden by any of the registered custom tests, so the argument is directly replaced by `TextScaler`. **To Be Deprecated**: The method/getter/setter/argument can't be deprecated in this PR because a registered customer test depends on it and a Flutter fix isn't available (or the test was run without applying flutter fixes first). This method/getter/setter/argument will be deprecated in a followup PR once the registered test is migrated. ### `Painting` Library | Affected API | State of `textScaleFactor` | Comment | | --- | --- | --- | | `InlineSpan.build({ double textScaleFactor = 1.0 })` argument | **Replaced** | | | `TextStyle.getParagraphStyle({ double TextScaleFactor = 1.0 })` argument | **Replaced** | | | `TextStyle.getTextStyle({ double TextScaleFactor = 1.0 })` argument| Deprecated | Can't replace: https://github.com/superlistapp/super_editor/blob/c47fd38dca4b7f43611690913b551a1773c563d7/super_editor/lib/src/infrastructure/super_textfield/desktop/desktop_textfield.dart#L1903-L1905| | `TextPainter({ double TextScaleFactor = 1.0 })` constructor argument | Deprecated | | | `TextPainter.textScaleFactor` getter and setter | Deprecated | No Flutter Fix, not expressible yet | | `TextPainter.computeWidth({ double TextScaleFactor = 1.0 })` argument | Deprecated | | | `TextPainter.computeMaxIntrinsicWidth({ double TextScaleFactor = 1.0 })` argument | Deprecated | | ### `Rendering` Library | Affected API | State of `textScaleFactor` | Comment | | --- | --- | --- | | `RenderEditable({ double TextScaleFactor = 1.0 })` constructor argument | Deprecated | | | `RenderEditable.textScaleFactor` getter and setter | Deprecated | No Flutter Fix, not expressible yet | | `RenderParagraph({ double TextScaleFactor = 1.0 })` constructor argument | Deprecated | | | `RenderParagraph.textScaleFactor` getter and setter | Deprecated | No Flutter Fix, not expressible yet | ### `Widgets` Library | Affected API | State of `textScaleFactor` | Comment | | --- | --- | --- | | `MediaQueryData({ double TextScaleFactor = 1.0 })` constructor argument | **To Be Deprecated** | https://github.com/flutter/packages/blob/cd7b93532e5cb605a42735e20f1de70fc00adae7/packages/flutter_markdown/test/text_scale_factor_test.dart#LL39C21-L39C35 | | `MediaQueryData.textScaleFactor` getter | Deprecated | | | `MediaQueryData.copyWith({ double? TextScaleFactor })` argument | Deprecated | | | `MediaQuery.maybeTextScaleFactorOf(BuildContext context)` static method | Deprecated | No Flutter Fix, not expressible yet | | `MediaQuery.textScaleFactorOf(BuildContext context)` static method | **To Be Deprecated** | https://github.com/flutter/packages/blob/cd7b93532e5cb605a42735e20f1de70fc00adae7/packages/flutter_markdown/lib/src/_functions_io.dart#L68-L70, No Flutter Fix, not expressible yet | | `RichText({ double TextScaleFactor = 1.0 })` constructor argument | **To Be Deprecated** | https://github.com/flutter/packages/blob/cd7b93532e5cb605a42735e20f1de70fc00adae7/packages/flutter_markdown/lib/src/builder.dart#L829-L843 | | `RichText.textScaleFactor` getter | **To Be Deprecated** | A constructor argument can't be deprecated right away| | `Text({ double? TextScaleFactor = 1.0 })` constructor argument | **To Be Deprecated** | https://github.com/flutter/packages/blob/914d120da12fba458c020210727831c31bd71041/packages/rfw/lib/src/flutter/core_widgets.dart#L647 , No Flutter Fix because of https://github.com/dart-lang/sdk/issues/52664 | | `Text.rich({ double? TextScaleFactor = 1.0 })` constructor argument | **To Be Deprecated** | The default constructor has an argument that can't be deprecated right away. No Flutter Fix because of https://github.com/dart-lang/sdk/issues/52664 | | `Text.textScaleFactor` getter | **To Be Deprecated** | A constructor argument can't be deprecated right away | | `EditableText({ double? TextScaleFactor = 1.0 })` constructor argument | Deprecated | No Flutter Fix because of https://github.com/dart-lang/sdk/issues/52664 | | `EditableText.textScaleFactor` getter | Deprecated | | ### `Material` Library | Affected API | State of `textScaleFactor` | Comment | | --- | --- | --- | | `SelectableText({ double? TextScaleFactor = 1.0 })` constructor argument | **To Be Deprecated** | https://github.com/flutter/packages/blob/cd7b93532e5cb605a42735e20f1de70fc00adae7/packages/flutter_markdown/lib/src/builder.dart#L829-L843, No Flutter Fix because of https://github.com/dart-lang/sdk/issues/52664 | | `SelectableText.rich({ double? TextScaleFactor = 1.0 })` constructor argument | **To Be Deprecated** | The default constructor has an argument that can't be deprecated right away. No Flutter Fix because of https://github.com/dart-lang/sdk/issues/52664 | | `SelectableText.textScaleFactor` getter | **To Be Deprecated** | A constructor argument can't be deprecated right away | A lot of material widgets (`Slider`, `RangeSlider`, `TimePicker`, and different types of buttons) also change their layout based on `textScaleFactor`. These need to be handled in a case-by-case fashion and will be migrated in follow-up PRs.
-
- 07 Jul, 2023 1 commit
-
-
Alexander Aprelev authored
Manual roll is needed because incoming dart sdk requires updated version vm_snapshot_analysis (>=0.7.4). https://github.com/flutter/engine/compare/5ae09b8b4fa381e8723ed5382a26eafd0d97236f...7c83ea3e854202aea22405a947ac1b8f18c5a72c ``` 7c83ea3e85 Reland "Manual roll Dart SDK from 2d98d9e27dae to 0b07debd5862 (21 revisions) (#43457)" (#43472) 9ef3e8d533 Roll Skia from 5eba922297bb to 93c92f97f5ab (2 revisions) (#43471) ``` Remove implementation of SuitePlatform from the test as well. Remove use of fake cwd from SuitePlatform as it can't be properly faked.
-
- 06 Jul, 2023 1 commit
-
-
Michael Goderbauer authored
PLUS: clean-up of all the unreachable stuff.
-
- 30 Jun, 2023 1 commit
-
-
Taha Tesser authored
fixes [NavigationDrawer selected item has wrong icon color [Material3 spec]](https://github.com/flutter/flutter/issues/129572) ### Description This PR fixes a mistake in the `NavigationDrawer` defaults, where generated token value returns a `null`. This issue can be detected when you want to customize the selected icon color for `NavigationDrawerDestination` using a custom color scheme. ### Code sample <details> <summary>expanded to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, themeMode: ThemeMode.light, theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.blue).copyWith( onSecondaryContainer: Colors.red, ), useMaterial3: true, ), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('NavigationDrawer Sample'), ), drawer: const NavigationDrawer( children: <Widget>[ NavigationDrawerDestination( icon: Icon(Icons.favorite_outline_rounded), label: Text('Favorite'), selectedIcon: Icon(Icons.favorite_rounded), ), NavigationDrawerDestination( icon: Icon(Icons.favorite_outline_rounded), label: Text('Favorite'), ), ], ), ); } } ``` </details> ### Before <img width="1053" alt="Screenshot 2023-06-27 at 13 24 38" src="https://github.com/flutter/flutter/assets/48603081/18c13a73-688f-4586-bb60-bddef45d173f"> ### After <img width="1053" alt="Screenshot 2023-06-27 at 13 24 25" src="https://github.com/flutter/flutter/assets/48603081/8a1427c6-517f-424a-b0bd-24bad7c5fbb0">
-
- 22 Jun, 2023 2 commits
-
-
Kate Lovett authored
Reverts flutter/flutter#125974
-
Taha Tesser authored
fix https://github.com/flutter/flutter/issues/117722 ### Description 1. Fix the divider doesn't stretch to take all the available width in the scrollable tab bar in M3 2. Add `dividerHeight` property. 3. Update the default tab alignment for the scrollable tab bar to match the specs (this is backward compatible for M2 with the new `tabAlignment` property). ### Bug (default tab alignment) 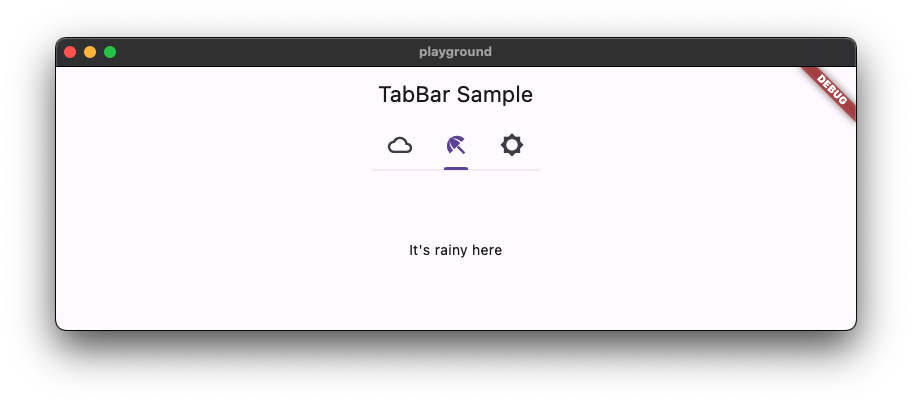 ### Fix (default tab alignment) 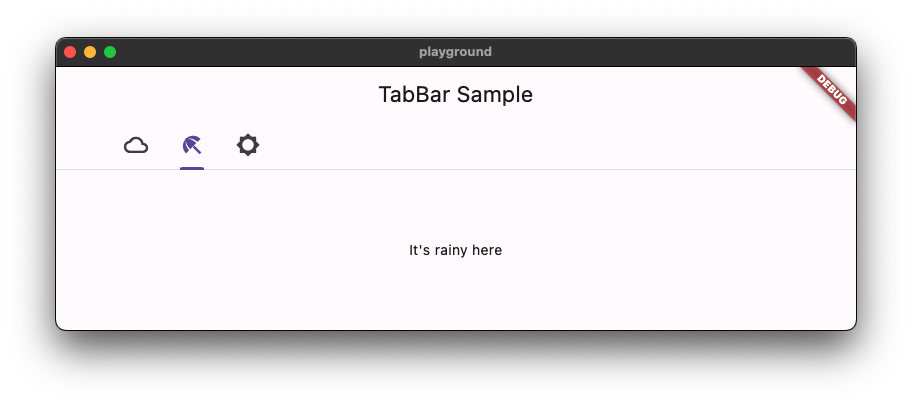 ### Code sample <details> <summary>code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [TabBar]. void main() => runApp(const TabBarApp()); class TabBarApp extends StatelessWidget { const TabBarApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( // tabBarTheme: const TabBarTheme(tabAlignment: TabAlignment.start), useMaterial3: true, ), home: const TabBarExample(), ); } } class TabBarExample extends StatefulWidget { const TabBarExample({super.key}); @override State<TabBarExample> createState() => _TabBarExampleState(); } class _TabBarExampleState extends State<TabBarExample> { bool rtl = false; @override Widget build(BuildContext context) { return DefaultTabController( initialIndex: 1, length: 3, child: Directionality( textDirection: rtl ? TextDirection.rtl : TextDirection.ltr, child: Scaffold( appBar: AppBar( title: const Text('TabBar Sample'), ), body: const Column( children: <Widget>[ Text('Scrollable-TabAlignment.start'), TabBar( isScrollable: true, tabAlignment: TabAlignment.start, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Text('Scrollable-TabAlignment.startOffset'), TabBar( isScrollable: true, tabAlignment: TabAlignment.startOffset, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Text('Scrollable-TabAlignment.center'), TabBar( isScrollable: true, tabAlignment: TabAlignment.center, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Spacer(), Text('Non-scrollable-TabAlignment.fill'), TabBar( tabAlignment: TabAlignment.fill, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Text('Non-scrollable-TabAlignment.center'), TabBar( tabAlignment: TabAlignment.center, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Spacer(), ], ), floatingActionButton: FloatingActionButton.extended( onPressed: () { setState(() { rtl = !rtl; }); }, label: const Text('Switch Direction'), icon: const Icon(Icons.swap_horiz), ), ), ), ); } } ``` </details> 
-