Fix `TimePicker` defaults for `hourMinuteTextStyle` and `dayPeriodTextColor`...
Fix `TimePicker` defaults for `hourMinuteTextStyle` and `dayPeriodTextColor` for Material 3 (#131253) fixes [`TimePicker` color and visual issues](https://github.com/flutter/flutter/issues/127035) ## Description - fixes default text style for `TimePicker`s `hourMinuteTextStyle` and added a todo for https://github.com/flutter/flutter/issues/131247 - fixes correct default color not being accessed for `dayPeriodTextColor` - Updates tests ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, theme: ThemeData(useMaterial3: true), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Sample'), ), body: Center( child: ElevatedButton( onPressed: () { showTimePicker( context: context, orientation: Orientation.portrait, initialEntryMode: TimePickerEntryMode.input, initialTime: TimeOfDay.now(), builder: (BuildContext context, Widget? child) { return MediaQuery( data: MediaQuery.of(context) .copyWith(alwaysUse24HourFormat: true), child: child!, ); }, ); }, child: const Text('Open Time Picker'), ), ), ); } } ``` </details> ### Before 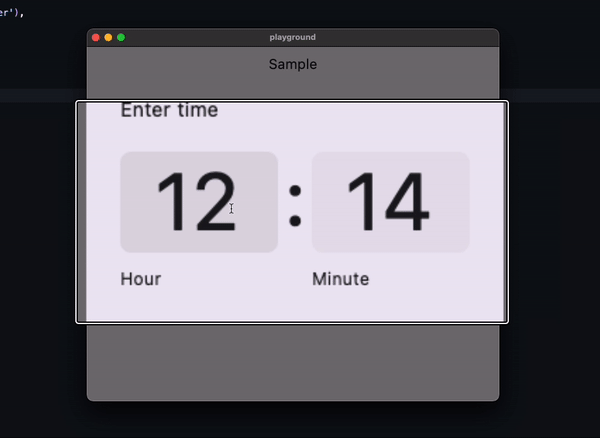 ### After 
Showing
Please register or sign in to comment