- 20 Sep, 2023 1 commit
-
-
Greg Spencer authored
## Description This removes all of the comments that are of the form "so-and-so (must not be null|can ?not be null|must be non-null)" from the cases where those values are defines as non-nullable values. This PR removes them from the material library. This was done by hand, since it really didn't lend itself to scripting, so it needs to be more than just spot-checked, I think. I was careful to leave any comment that referred to parameters that were nullable, but I may have missed some. In addition to being no longer relevant after null safety has been made the default, these comments were largely fragile, in that it was easy for them to get out of date, and not be accurate anymore anyhow. This did create a number of constructor comments which basically say "Creates a [Foo].", but I don't really know how to avoid that in a large scale change, since there's not much you can really say in a lot of cases. I think we might consider some leniency for constructors to the "Comment must be meaningful" style guidance (which we de facto have already, since there are a bunch of these). ## Related PRs - https://github.com/flutter/flutter/pull/134984 - https://github.com/flutter/flutter/pull/134992 - https://github.com/flutter/flutter/pull/134993 - https://github.com/flutter/flutter/pull/134994 ## Tests - Documentation only change.
-
- 06 Sep, 2023 1 commit
-
-
Tirth authored
Adds parent prop `onTap` to CheckedPopupMenuItem. Fixes #127800
-
- 25 Aug, 2023 1 commit
-
-
Taha Tesser authored
fixes [`PopupMenuItem` with a `ListTile` doesn't use the correct text style.](https://github.com/flutter/flutter/issues/133138) ### Description This fixes an issue text style issue for `PopupMenuItem` with a `ListTile` (for an elaborate popup menu) https://api.flutter.dev/flutter/material/PopupMenuItem-class.html <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [PopupMenuButton]. // This is the type used by the popup menu below. enum SampleItem { itemOne, itemTwo, itemThree } void main() => runApp(const PopupMenuApp()); class PopupMenuApp extends StatelessWidget { const PopupMenuApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( useMaterial3: true, textTheme: const TextTheme( labelLarge: TextStyle( fontStyle: FontStyle.italic, fontWeight: FontWeight.bold, ), ), ), home: const PopupMenuExample(), ); } } class PopupMenuExample extends StatefulWidget { const PopupMenuExample({super.key}); @override State<PopupMenuExample> createState() => _PopupMenuExampleState(); } class _PopupMenuExampleState extends State<PopupMenuExample> { SampleItem? selectedMenu; @override Widget build(BuildContext context) { return Scaffold( body: Center( child: SizedBox( width: 300, height: 130, child: Align( alignment: Alignment.topLeft, child: PopupMenuButton<SampleItem>( initialValue: selectedMenu, // Callback that sets the selected popup menu item. onSelected: (SampleItem item) { setState(() { selectedMenu = item; }); }, itemBuilder: (BuildContext context) => <PopupMenuEntry<SampleItem>>[ const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: Text('PopupMenuItem'), ), const CheckedPopupMenuItem<SampleItem>( checked: true, value: SampleItem.itemTwo, child: Text('CheckedPopupMenuItem'), ), const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: ListTile( leading: Icon(Icons.cloud), title: Text('ListTile'), contentPadding: EdgeInsets.zero, trailing: Icon(Icons.arrow_right_rounded), ), ), ], ), ), ), ), ); } } ``` </details> ### Before  ### After 
-
- 16 Aug, 2023 1 commit
-
-
Taha Tesser authored
This relands https://github.com/flutter/flutter/pull/131609 --- fixes [`PopupMenuItem` adds redundant padding when using `ListItem`](https://github.com/flutter/flutter/issues/128553)
-
- 13 Aug, 2023 1 commit
-
-
Casey Hillers authored
Revert "Fix `PopupMenuItem` & `CheckedPopupMenuItem` has redundant `ListTile` padding and update default horizontal padding for Material 3" (#132457) Reverts flutter/flutter#131609 b/295497265 - This broke Google Testing. We'll need the internal patch before landing as there's a large number of customer codebases impacted.
-
- 10 Aug, 2023 1 commit
-
-
Taha Tesser authored
Fix `PopupMenuItem` & `CheckedPopupMenuItem` has redundant `ListTile` padding and update default horizontal padding for Material 3 (#131609) fixes [`PopupMenuItem` adds redundant padding when using `ListItem`](https://github.com/flutter/flutter/issues/128553) ### Description - Fixed redundant `ListTile` padding when using `CheckedPopupMenuItem` or `PopupMenuItem` with the `ListTile` child for complex popup menu items as suggested in the docs. - Updated default horizontal padding for popup menu items. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [PopupMenuButton]. // This is the type used by the popup menu below. enum SampleItem { itemOne, itemTwo, itemThree } void main() => runApp(const PopupMenuApp()); class PopupMenuApp extends StatelessWidget { const PopupMenuApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData(useMaterial3: true), home: const PopupMenuExample(), ); } } class PopupMenuExample extends StatefulWidget { const PopupMenuExample({super.key}); @override State<PopupMenuExample> createState() => _PopupMenuExampleState(); } class _PopupMenuExampleState extends State<PopupMenuExample> { SampleItem? selectedMenu; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: const Text('PopupMenuButton')), body: Center( child: SizedBox( width: 150, height: 100, child: Align( alignment: Alignment.topLeft, child: PopupMenuButton<SampleItem>( initialValue: selectedMenu, // Callback that sets the selected popup menu item. onSelected: (SampleItem item) { setState(() { selectedMenu = item; }); }, itemBuilder: (BuildContext context) => <PopupMenuEntry<SampleItem>>[ const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: Text('PopupMenuItem'), ), const CheckedPopupMenuItem<SampleItem>( checked: true, value: SampleItem.itemTwo, child: Text('CheckedPopupMenuItem'), ), const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: ListTile( leading: Icon(Icons.cloud), title: Text('ListTile'), contentPadding: EdgeInsets.zero, trailing: Icon(Icons.arrow_right_rounded), ), ), ], ), ), ), ), ); } } ``` </details> ### Before  - Default horizontal padding is the same as M2 (16.0), while the specs use a smaller value (12.0) - `ListTile` nested by default in `CheckedPopupMenuItem` has redundant padding - `PopupMenuItem` using `ListTile` as a child for complex menu items contains redundant padding.  ### After - Default horizontal padding is updated for Material 3. - `PopupMenuItem` & `CheckedPopupMenuItem` override `ListTile` padding (similar to how `ExpansionTile` overrides `ListTile` text and icon color. 
-
- 08 Aug, 2023 1 commit
-
-
Taha Tesser authored
Add `PopupMenuButton.iconColor`, `PopupMenuTheme.iconSize` and fix button icon using unexpected color propert (#132054) fixes [PopupMenuButton uses color property for icon color](https://github.com/flutter/flutter/issues/127802) fixes [`popup_menu_test.dart` lacks default icon color tests.](https://github.com/flutter/flutter/issues/132050) ### Description - Add `PopupMenuButton..iconColor` and fix the PopupMenu button icon using an unexpected color property. - Add the missing `PopupMenuTheme.iconSize`. - Clean up some tests and minor improvements. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [PopupMenuButton]. // This is the type used by the popup menu below. enum SampleItem { itemOne, itemTwo, itemThree } void main() => runApp(const PopupMenuApp()); class PopupMenuApp extends StatelessWidget { const PopupMenuApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( popupMenuTheme: PopupMenuThemeData( // iconSize: 75, // iconColor: Colors.amber, color: Colors.deepPurple[100], ), ), home: const PopupMenuExample(), ); } } class PopupMenuExample extends StatefulWidget { const PopupMenuExample({super.key}); @override State<PopupMenuExample> createState() => _PopupMenuExampleState(); } class _PopupMenuExampleState extends State<PopupMenuExample> { SampleItem? selectedMenu; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: const Text('PopupMenuButton')), body: Center( child: PopupMenuButton<SampleItem>( iconSize: 75, // iconColor: Colors.amber, color: Colors.deepPurple[100], initialValue: selectedMenu, // Callback that sets the selected popup menu item. onSelected: (SampleItem item) { setState(() { selectedMenu = item; }); }, itemBuilder: (BuildContext context) => <PopupMenuEntry<SampleItem>>[ const PopupMenuItem<SampleItem>( value: SampleItem.itemOne, child: Text('Item 1'), ), const PopupMenuItem<SampleItem>( value: SampleItem.itemTwo, child: Text('Item 2'), ), const PopupMenuDivider(), const CheckedPopupMenuItem<SampleItem>( value: SampleItem.itemThree, checked: true, child: Text('Item 3'), ), ], ), ), ); } } ``` </details>  
-
- 28 Jul, 2023 1 commit
-
-
Taha Tesser authored
fixes [Update `CheckedPopupMenuItemâ` for Material 3](https://github.com/flutter/flutter/issues/128576) ### Description - This adds the missing ``CheckedPopupMenuItemâ.labelTextStyle` parameter - Fixes default text style for `CheckedPopupMenuItemâ`. It used `ListTile`'s `bodyLarge` instead of `LabelLarge` similar to `PopupMenuItem`. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, theme: ThemeData( useMaterial3: true, textTheme: const TextTheme( labelLarge: TextStyle( fontWeight: FontWeight.bold, fontStyle: FontStyle.italic, letterSpacing: 5.0, ), ), ), home: const Example(), ); } } class Example extends StatelessWidget { const Example({super.key}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Sample'), actions: <Widget>[ PopupMenuButton<String>( icon: const Icon(Icons.more_vert), itemBuilder: (BuildContext context) => <PopupMenuEntry<String>>[ const CheckedPopupMenuItem<String>( // labelTextStyle: MaterialStateProperty.resolveWith( // (Set<MaterialState> states) { // if (states.contains(MaterialState.selected)) { // return const TextStyle( // color: Colors.red, // fontStyle: FontStyle.italic, // fontWeight: FontWeight.bold, // ); // } // return const TextStyle( // color: Colors.amber, // fontStyle: FontStyle.italic, // fontWeight: FontWeight.bold, // ); // }), child: Text('Mild'), ), const CheckedPopupMenuItem<String>( checked: true, // labelTextStyle: MaterialStateProperty.resolveWith( // (Set<MaterialState> states) { // if (states.contains(MaterialState.selected)) { // return const TextStyle( // color: Colors.red, // fontStyle: FontStyle.italic, // fontWeight: FontWeight.bold, // ); // } // return const TextStyle( // color: Colors.amber, // fontStyle: FontStyle.italic, // fontWeight: FontWeight.bold, // ); // }), child: Text('Spicy'), ), const PopupMenuDivider(), const PopupMenuItem<String>( value: 'Close', child: Text('Close'), ), ], ) ], ), ); } } ``` </details> ### Customized `textTheme.labelLarge` text theme. | Before | After | | --------------- | --------------- | | <img src="https://github.com/flutter/flutter/assets/48603081/2672438d-b2da-479b-a5d3-d239ef646365" /> | <img src="https://github.com/flutter/flutter/assets/48603081/b9f83719-dede-4c2f-8247-18f74e63eb29" /> | ### New `CheckedPopupMenuItemâ.labelTextStyle` parameter with material states support <img src="https://github.com/flutter/flutter/assets/48603081/ef0a88aa-9811-42b1-a3aa-53b90c8d43fb" height="450" />
-
- 19 Jul, 2023 1 commit
-
-
Greg Spencer authored
## Description Modifies the semantic label for popup and context menus to be "Dismiss menu" instead of just "Dismiss". ## Related Issues - Fixes https://github.com/flutter/flutter/issues/118994 ## Tests - Updated tests
-
- 09 Jun, 2023 1 commit
-
-
Pierre-Louis authored
## Description This improves defaults generation with logging, stats, and token validation. This PR includes these changes: * introduce `TokenLogger`, with a verbose mode * prints versions and tokens usage to the console * outputs `generated/used_tokens.csv`, a list of all used tokens, for use by Google * find token files in `data` automatically * hide tokens `Map` * tokens can be obtained using existing resolvers (e.g. `color`, `shape`), or directly through `getToken`. * tokens can be checked for existence with `tokenAvailable` * remove version from template, since the tokens are aggregated and multiple versions are possible (as is the case currently), it does not make sense to attribute a single version * improve documentation ## Related Issues - Fixes https://github.com/flutter/flutter/issues/122602 ## Tests - Added tests for `TokenLogger` - Regenerated tokens, no-op except version removal ## Future work A future PR should replace or remove the following invalid tokens usages <img width="578" alt="image" src="https://github.com/flutter/flutter/assets/6655696/b6f9e5a7-523f-4f72-94f9-1b0bf4cc9f00">
-
- 08 Jun, 2023 1 commit
-
-
Mahdi Bagheri authored
*The order of calling Navigator.pop and PopupMenuItem.onTap has been changed so before calling PopupMenuItem onTap method, PopupMenuBotton onSelect method is going to be called.* *Solves #127443* *If you had to change anything in the [flutter/tests] repo, include a link to the migration guide as per the [breaking change policy].*
-
- 07 Jun, 2023 1 commit
-
-
Leigha Jarett authored
Fixes: https://github.com/flutter/flutter/issues/127215
-
- 02 May, 2023 1 commit
-
-
Valentin Vignal authored
Align the `PopupMenu` under its child. Before: 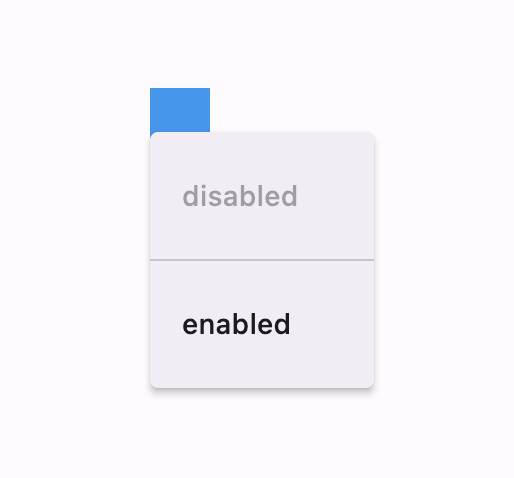 After: 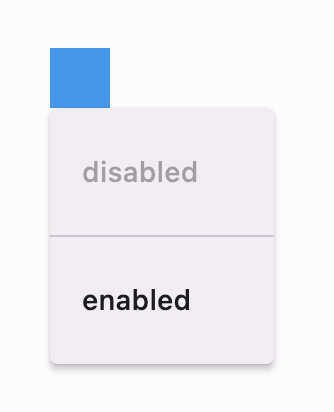 *List which issues are fixed by this PR. You must list at least one issue.* Fixes https://github.com/flutter/flutter/issues/125474 *If you had to change anything in the [flutter/tests] repo, include a link to the migration guide as per the [breaking change policy].*
-
- 28 Apr, 2023 1 commit
-
-
fzyzcjy authored
Background: I am adding logging to things like dialogs, bottom sheets and menus. Then I realized that, showMenu does not allow users to provide RouteSettings, while showDialog and showModalBottomSheet both allow. Therefore, IMHO a consistent API design may need to add this to showMenu. I will add tests if this proposal looks OK :)
-
- 22 Mar, 2023 1 commit
-
-
Michael Goderbauer authored
Remove 1745 decorative breaks
-
- 13 Mar, 2023 1 commit
-
-
Pierre-Louis authored
Update Material tokens to 0.162
-
- 17 Feb, 2023 1 commit
-
-
Ian Hickson authored
* lerp documentation * Remove Note, Note That from repo * Improve BorderSide documentation. * apply review comments
-
- 07 Feb, 2023 1 commit
-
-
Qun Cheng authored
* Update to v0.158 of the token database. * Update checkbox template * Fix DatePickerTheme test --------- Co-authored-by:
Qun Cheng <quncheng@google.com>
-
- 24 Jan, 2023 1 commit
-
-
Michael Goderbauer authored
* dart fix --apply * manual fixes
-
- 17 Jan, 2023 1 commit
-
-
Darren Austin authored
-
- 10 Jan, 2023 1 commit
-
-
Yegor authored
* allow focus to leave FlutterView * fix tests and docs * small doc update * fix analysis lint * use closed loop for dialogs * add tests for new API * address comments * test FocusScopeNode.traversalEdgeBehavior setter; reverse wrap-around * rename actionResult to invokeResult * address comments
-
- 03 Jan, 2023 1 commit
-
-
Darren Austin authored
* Updated to tokens v0.150. * Updated with a reverted list_tile.dart.
-
- 20 Dec, 2022 1 commit
-
-
Michael Goderbauer authored
-
- 09 Dec, 2022 1 commit
-
-
Callum Moffat authored
-
- 28 Nov, 2022 1 commit
-
-
Darren Austin authored
-
- 15 Nov, 2022 1 commit
-
-
Darren Austin authored
-
- 01 Nov, 2022 2 commits
-
-
Taha Tesser authored
-
Darren Austin authored
-
- 24 Oct, 2022 1 commit
-
-
Taha Tesser authored
-
- 06 Sep, 2022 1 commit
-
-
Valentin Vignal authored
-
- 22 Aug, 2022 1 commit
-
-
Kate Lovett authored
-
- 14 Aug, 2022 1 commit
-
-
Ademir Villena authored
-
- 03 Aug, 2022 1 commit
-
-
Qun Cheng authored
* Created IconButtonTheme and apply it to IconButton
-
- 12 Jul, 2022 1 commit
-
-
Taha Tesser authored
-
- 03 Jun, 2022 1 commit
-
-
Taha Tesser authored
-
- 25 May, 2022 1 commit
-
-
Pierre-Louis authored
* Use `curly_braces_in_flow_control_structures` for `material` * include test/material * add back removed comments
-
- 18 May, 2022 1 commit
-
-
gaaclarke authored
-
- 27 Apr, 2022 1 commit
-
-
Pierre-Louis authored
-
- 14 Apr, 2022 1 commit
-
-
Michael Goderbauer authored
-
- 28 Mar, 2022 1 commit
-
-
Andrei Diaconu authored
-