- 28 Feb, 2024 1 commit
-
-
Qun Cheng authored
-
- 27 Feb, 2024 2 commits
-
-
auto-submit[bot] authored
Reverts "Reland - Introduce tone-based surfaces and accent color add-ons - Part 2 (#144001)" (#144262) Reverts flutter/flutter#144001 Initiated by: Piinks Reason for reverting: Failing goldens at the tip of tree Original PR Author: QuncCccccc Reviewed By: {HansMuller} This change reverts the following previous change: Original Description: Reverts flutter/flutter#143973 This is a reland for #138521 with an updated g3fix(cl/605555997). Local test: cl/609608958.
-
Qun Cheng authored
Reverts flutter/flutter#143973 This is a reland for #138521 with an updated g3fix(cl/605555997). Local test: cl/609608958.
-
- 22 Feb, 2024 1 commit
-
-
Qun Cheng authored
Reverts flutter/flutter#138521
-
- 20 Feb, 2024 1 commit
-
-
Qun Cheng authored
This PR is to introduce 19 new color roles and deprecate 3 color roles in `ColorScheme`. **Tone-based surface colors** (7 colors): * surfaceBright * surfaceDim * surfaceContainer * surfaceContainerLowest * surfaceContainerLow * surfaceContainerHigh * surfaceContainerHighest **Accent color add-ons** (12 colors): * primary/secondary/tertiary-Fixed * primary/secondary/tertiary-FixedDim * onPrimary/onSecondary/onTertiary-Fixed * onPrimary/onSecondary/onTertiary-FixedVariant **Deprecated colors**: * background -> replaced with surface * onBackground -> replaced with onSurface * surfaceVariant -> replaced with surfaceContainerHighest Please checkout this [design doc](https://docs.google.com/document/d/1ODqivpM_6c490T4j5XIiWCDKo5YqHy78YEFqDm4S8h4/edit?usp=sharing) for more information:) 
-
- 18 Jan, 2024 1 commit
-
-
David Martos authored
This PR improves the distance between the label and the icon in the Tab widget. I updated the margin to 2 pixels, taken from the Figma design page for Material 3. On Material 2 I left the default value of 10 pixels. Related to #128696 (In particular, the distance between label and icon) Here are some screenshots for comparison. I looked a bit into the other mentioned issue of the tab height not following the M3 spec. Flutter uses 72 and the spec uses 64. But because Tab is a PreferredSizeWidget, I don't think there is an easy way to provide a different size depending on `ThemeData.useMaterial3`, because there is no `BuildContext` available. I provide a sample image for the 64 height as well for context on the linked issue, even though it's not part of the PR changes. The screenshots are taken side by side with the image at: https://m3.material.io/components/tabs/guidelines ## Original  ## New (tab height = 72, Flutter default for 8 years)  ## New (tab height = 64, M3 spec) 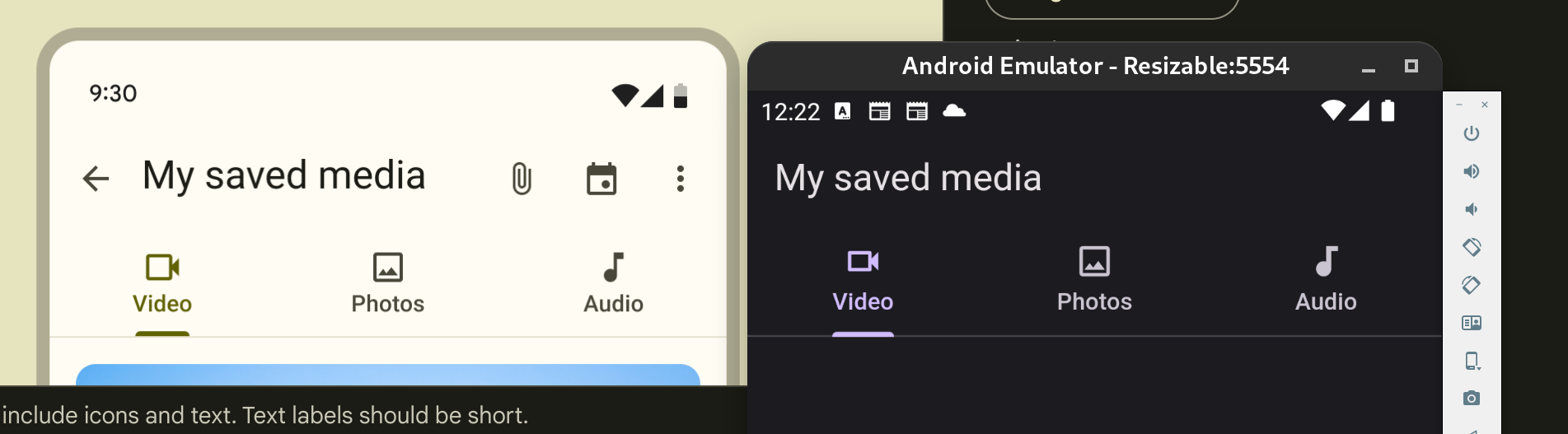
-
- 02 Aug, 2023 1 commit
-
-
Taha Tesser authored
fixes [Material 3 `TabBar` does not take full width when `isScrollable: true`](https://github.com/flutter/flutter/issues/117722) ### Description 1. Fixed the divider doesn't stretch to take all the available width in the scrollable tab bar in M3 2. Added `dividerHeight` property. ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [TabBar]. void main() => runApp(const TabBarApp()); class TabBarApp extends StatelessWidget { const TabBarApp({super.key}); @override Widget build(BuildContext context) { return const MaterialApp( debugShowCheckedModeBanner: false, home: TabBarExample(), ); } } class TabBarExample extends StatefulWidget { const TabBarExample({super.key}); @override State<TabBarExample> createState() => _TabBarExampleState(); } class _TabBarExampleState extends State<TabBarExample> { bool rtl = false; bool customColors = false; bool removeDivider = false; Color dividerColor = Colors.amber; Color indicatorColor = Colors.red; @override Widget build(BuildContext context) { return DefaultTabController( initialIndex: 1, length: 3, child: Directionality( textDirection: rtl ? TextDirection.rtl : TextDirection.ltr, child: Scaffold( appBar: AppBar( title: const Text('TabBar Sample'), actions: <Widget>[ IconButton.filledTonal( tooltip: 'Switch direction', icon: const Icon(Icons.swap_horiz), onPressed: () { setState(() { rtl = !rtl; }); }, ), IconButton.filledTonal( tooltip: 'Use custom colors', icon: const Icon(Icons.color_lens), onPressed: () { setState(() { customColors = !customColors; }); }, ), IconButton.filledTonal( tooltip: 'Show/hide divider', icon: const Icon(Icons.remove_rounded), onPressed: () { setState(() { removeDivider = !removeDivider; }); }, ), ], ), body: Column( children: <Widget>[ const Spacer(), const Text('Scrollable - TabAlignment.start'), TabBar( isScrollable: true, tabAlignment: TabAlignment.start, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Scrollable - TabAlignment.startOffset'), TabBar( isScrollable: true, tabAlignment: TabAlignment.startOffset, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Scrollable - TabAlignment.center'), TabBar( isScrollable: true, tabAlignment: TabAlignment.center, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Spacer(), const Text('Non-scrollable - TabAlignment.fill'), TabBar( tabAlignment: TabAlignment.fill, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Non-scrollable - TabAlignment.center'), TabBar( tabAlignment: TabAlignment.center, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Spacer(), const Text('Secondary - TabAlignment.fill'), TabBar.secondary( tabAlignment: TabAlignment.fill, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Text('Secondary - TabAlignment.center'), TabBar.secondary( tabAlignment: TabAlignment.center, dividerColor: customColors ? dividerColor : null, indicatorColor: customColors ? indicatorColor : null, dividerHeight: removeDivider ? 0 : null, tabs: const <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), const Spacer(), ], ), ), ), ); } } ``` </details> ### Before  ### After  This also contains regression test for https://github.com/flutter/flutter/pull/125974#discussion_r1239089151 ```dart // This is a regression test for https://github.com/flutter/flutter/pull/125974#discussion_r1239089151. testWidgets('Divider can be constrained', (WidgetTester tester) async { ``` 
-
- 22 Jun, 2023 2 commits
-
-
Kate Lovett authored
Reverts flutter/flutter#125974
-
Taha Tesser authored
fix https://github.com/flutter/flutter/issues/117722 ### Description 1. Fix the divider doesn't stretch to take all the available width in the scrollable tab bar in M3 2. Add `dividerHeight` property. 3. Update the default tab alignment for the scrollable tab bar to match the specs (this is backward compatible for M2 with the new `tabAlignment` property). ### Bug (default tab alignment) 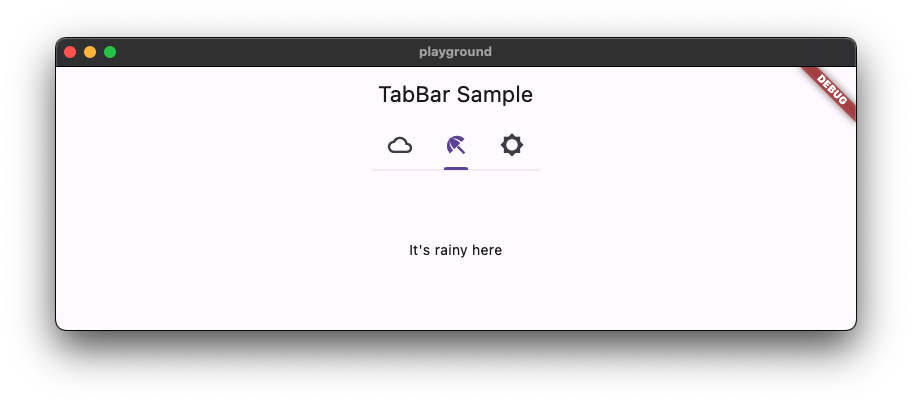 ### Fix (default tab alignment) 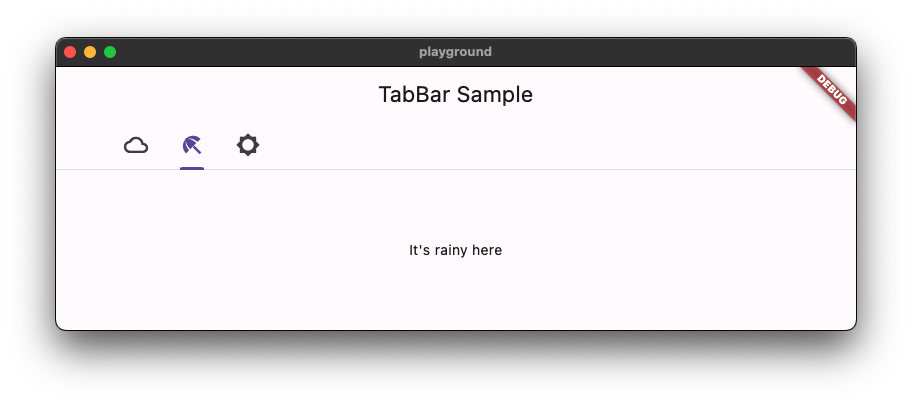 ### Code sample <details> <summary>code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [TabBar]. void main() => runApp(const TabBarApp()); class TabBarApp extends StatelessWidget { const TabBarApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( // tabBarTheme: const TabBarTheme(tabAlignment: TabAlignment.start), useMaterial3: true, ), home: const TabBarExample(), ); } } class TabBarExample extends StatefulWidget { const TabBarExample({super.key}); @override State<TabBarExample> createState() => _TabBarExampleState(); } class _TabBarExampleState extends State<TabBarExample> { bool rtl = false; @override Widget build(BuildContext context) { return DefaultTabController( initialIndex: 1, length: 3, child: Directionality( textDirection: rtl ? TextDirection.rtl : TextDirection.ltr, child: Scaffold( appBar: AppBar( title: const Text('TabBar Sample'), ), body: const Column( children: <Widget>[ Text('Scrollable-TabAlignment.start'), TabBar( isScrollable: true, tabAlignment: TabAlignment.start, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Text('Scrollable-TabAlignment.startOffset'), TabBar( isScrollable: true, tabAlignment: TabAlignment.startOffset, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Text('Scrollable-TabAlignment.center'), TabBar( isScrollable: true, tabAlignment: TabAlignment.center, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Spacer(), Text('Non-scrollable-TabAlignment.fill'), TabBar( tabAlignment: TabAlignment.fill, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Text('Non-scrollable-TabAlignment.center'), TabBar( tabAlignment: TabAlignment.center, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), ), Tab( icon: Icon(Icons.beach_access_sharp), ), Tab( icon: Icon(Icons.brightness_5_sharp), ), ], ), Spacer(), ], ), floatingActionButton: FloatingActionButton.extended( onPressed: () { setState(() { rtl = !rtl; }); }, label: const Text('Switch Direction'), icon: const Icon(Icons.swap_horiz), ), ), ), ); } } ``` </details> 
-
- 09 Jun, 2023 1 commit
-
-
Pierre-Louis authored
## Description This improves defaults generation with logging, stats, and token validation. This PR includes these changes: * introduce `TokenLogger`, with a verbose mode * prints versions and tokens usage to the console * outputs `generated/used_tokens.csv`, a list of all used tokens, for use by Google * find token files in `data` automatically * hide tokens `Map` * tokens can be obtained using existing resolvers (e.g. `color`, `shape`), or directly through `getToken`. * tokens can be checked for existence with `tokenAvailable` * remove version from template, since the tokens are aggregated and multiple versions are possible (as is the case currently), it does not make sense to attribute a single version * improve documentation ## Related Issues - Fixes https://github.com/flutter/flutter/issues/122602 ## Tests - Added tests for `TokenLogger` - Regenerated tokens, no-op except version removal ## Future work A future PR should replace or remove the following invalid tokens usages <img width="578" alt="image" src="https://github.com/flutter/flutter/assets/6655696/b6f9e5a7-523f-4f72-94f9-1b0bf4cc9f00">
-
- 11 May, 2023 1 commit
-
-
Qun Cheng authored
Fixes #122250. This PR is to make sure all the MaterialStateProperty defaults are able to correctly resolve different states. * When a widget is pressed, it is also hovered, so we need to put the `MaterialState.pressed` check before `MaterialState.hovered`. * When a widget is focused, the widget should still be able to be hovered, so we should check `MaterialState.hovered` before `MaterialState.focused`. * There are also cases like in _InputDecoratorDefaultsM3, the `MaterialState.disabled` should be checked before `MaterialState.error`. the order should be disabled, (error), pressed, hovered, focused.
-
- 05 May, 2023 1 commit
-
-
Taha Tesser authored
fixes https://github.com/flutter/flutter/issues/123112 ### Description 1. Add proper M3 indicator height aka`IndictorWeight` from the M3 specs for the primary tab bar with label indicator size. https://github.com/flutter/flutter/blob/db6074ade4e4fde664e6258d671faf356e1b6e85/dev/tools/gen_defaults/data/navigation_tab_primary.json#L9 (this was held due to `indicatorWeight` having a hard-coded value) and added a secondary tab bar indicator height. 2. Set a minimum value for the rounded indicator to maintain the indicator shape. 3. With proper indicator height, the rounded indicator position is also fixed. 4. Fix round indicator is shown for the primary tab bar with tab indicator size. 5. Above changes fix https://github.com/flutter/flutter/issues/123112. 6. Fix the `startOffset` const value from https://github.com/flutter/flutter/pull/125036 to match docs and move it to a variable.
-
- 01 May, 2023 1 commit
-
-
Taha Tesser authored
fixes https://github.com/flutter/flutter/issues/124195 This introduces `TabBar.tabAlignment` while keeping the default alignment for both M2 and M3.
-
- 28 Mar, 2023 1 commit
-
-
Kate Lovett authored
Revert "Fix divider width in scrollable `TabBar` for Material 3 and add `dividerHeight` parameter (#123127)" (#123616) Revert "Fix divider width in scrollable `TabBar` for Material 3 and add `dividerHeight` parameter"
-
- 27 Mar, 2023 1 commit
-
-
Taha Tesser authored
Fix divider width in scrollable `TabBar` for Material 3 and add `dividerHeight` parameter (#123127) Fix divider width in scrollable `TabBar` for Material 3 and add `dividerHeight` parameter
-
- 23 Mar, 2023 1 commit
-
-
Pierre-Louis authored
Add support for secondary tab bar
-
- 30 Nov, 2022 2 commits
-
-
Taha Tesser authored
* Add Material 3 support for `TabBar` * M3 `TabBar` revert fix and tests
-
Pierre-Louis authored
This reverts commit 900b3954.
-
- 29 Nov, 2022 1 commit
-
-
Taha Tesser authored
-