Fix `TabBar` doesn't use `labelStyle` & `unselectedLabelStyle` color (#133989)
Fixes [TabBar labelStyle.color and unselectedLabelStyle.color does not take effect](https://github.com/flutter/flutter/issues/109484) ### Code sample <details> <summary>expand to view the code sample</summary> ```dart import 'package:flutter/material.dart'; /// Flutter code sample for [TabBar]. const Color labelColor = Color(0xFFFF0000); const Color unselectedLabelColor = Color(0x95FF0000); const TextStyle labelStyle = TextStyle( color: Color(0xff0000ff), fontWeight: FontWeight.bold, ); const TextStyle unselectedLabelStyle = TextStyle( color: Color(0x950000ff), fontStyle: FontStyle.italic, ); void main() => runApp(const TabBarApp()); class TabBarApp extends StatelessWidget { const TabBarApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData(useMaterial3: true), home: const TabBarExample(), ); } } class TabBarExample extends StatelessWidget { const TabBarExample({super.key}); @override Widget build(BuildContext context) { return DefaultTabController( initialIndex: 1, length: 3, child: Scaffold( appBar: AppBar( title: const Text('TabBar Sample'), bottom: const TabBar( // labelColor: labelColor, // unselectedLabelColor: unselectedLabelColor, labelStyle: labelStyle, unselectedLabelStyle: unselectedLabelStyle, tabs: <Widget>[ Tab( icon: Icon(Icons.cloud_outlined), text: 'Cloudy', ), Tab( icon: Icon(Icons.beach_access_sharp), text: 'Sunny', ), Tab( icon: Icon(Icons.brightness_5_sharp), text: 'Rainy', ), ], ), ), body: const TabBarView( children: <Widget>[ Center( child: Text("It's cloudy here"), ), Center( child: Text("It's rainy here"), ), Center( child: Text("It's sunny here"), ), ], ), ), ); } } ``` </details> #### When `labelStyle` and `unselectedLabelStyle` are specified with a color. ### Before  ### After 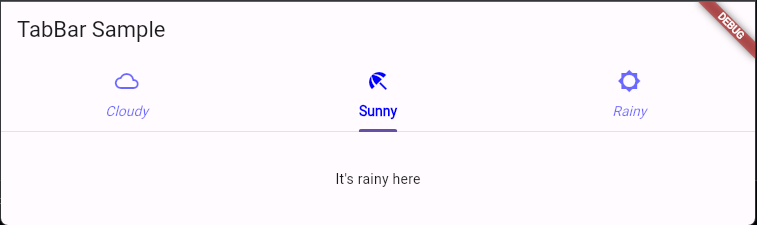
Showing
Please register or sign in to comment