Skip to content
Projects
Groups
Snippets
Help
Loading...
Help
Submit feedback
Sign in
Toggle navigation
F
Front-End
Project
Project
Details
Activity
Releases
Cycle Analytics
Repository
Repository
Files
Commits
Branches
Tags
Contributors
Graph
Compare
Charts
Issues
0
Issues
0
List
Board
Labels
Milestones
Merge Requests
0
Merge Requests
0
CI / CD
CI / CD
Pipelines
Jobs
Schedules
Charts
Wiki
Wiki
Snippets
Snippets
Members
Members
Collapse sidebar
Close sidebar
Activity
Graph
Charts
Create a new issue
Jobs
Commits
Issue Boards
Open sidebar
abdullh.alsoleman
Front-End
Commits
c8af729d
Unverified
Commit
c8af729d
authored
Sep 03, 2019
by
Kate Lovett
Committed by
GitHub
Sep 03, 2019
Browse files
Options
Browse Files
Download
Email Patches
Plain Diff
Golden Doc Updates (#39082)
parent
0ed3242a
Changes
2
Hide whitespace changes
Inline
Side-by-side
Showing
2 changed files
with
102 additions
and
18 deletions
+102
-18
goldens.dart
packages/flutter_test/lib/src/goldens.dart
+61
-4
matchers.dart
packages/flutter_test/lib/src/matchers.dart
+41
-14
No files found.
packages/flutter_test/lib/src/goldens.dart
View file @
c8af729d
...
...
@@ -23,6 +23,33 @@ import 'package:test_api/test_api.dart' as test_package show TestFailure;
/// fake async constraints that are normally imposed on widget tests (i.e. the
/// need or the ability to call [WidgetTester.pump] to advance the microtask
/// queue).
///
/// ## What is Golden File Testing?
///
/// The term __golden file__ refers to a master image that is considered the true
/// rendering of a given widget, state, application, or other visual
/// representation you have chosen to capture.
///
/// By keeping a master reference of visual aspects of your application, you can
/// prevent unintended changes as you develop by testing against them.
///
/// Here, a minor code change has altered the appearance of a widget. A golden
/// file test has compared the image generated at the time of the test to the
/// golden master file that was generated earlier. The test has identified the
/// change, preventing unintended modifications.
///
/// | Sample | Image |
/// |--------------------------------|--------|
/// | Golden Master Image | 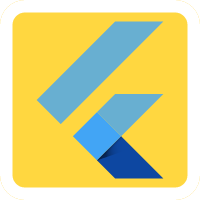 |
/// | Difference | 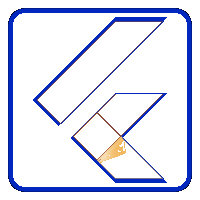 |
/// | Test image after modification | 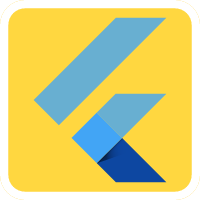 |
///
/// See also:
///
/// * [LocalFileComparator] for the default [GoldenFileComparator]
/// implementation for `flutter test`.
/// * [matchesGoldenFile], the function from [flutter_test] that invokes the
/// comparator.
abstract
class
GoldenFileComparator
{
/// Compares the pixels of decoded png [imageBytes] against the golden file
/// identified by [golden].
...
...
@@ -258,14 +285,44 @@ class TrivialComparator implements GoldenFileComparator {
/// The default [GoldenFileComparator] implementation for `flutter test`.
///
/// This comparator loads golden files from the local file system, treating the
/// golden key as a relative path from the test file's directory.
/// The term __golden file__ refers to a master image that is considered the true
/// rendering of a given widget, state, application, or other visual
/// representation you have chosen to capture. This comparator loads golden
/// files from the local file system, treating the golden key as a relative
/// path from the test file's directory.
///
/// This comparator performs a pixel-for-pixel comparison of the decoded PNGs,
/// returning true only if there's an exact match.
/// returning true only if there's an exact match. In cases where the captured
/// test image does not match the golden file, this comparator will provide
/// output to illustrate the difference, described in further detail below.
///
/// When using `flutter test --update-goldens`, [LocalFileComparator]
/// updates the files on disk to match the rendering.
/// updates the golden files on disk to match the rendering.
///
/// ## Local Output from Golden File Testing
///
/// The [LocalFileComparator] will output test feedback when a golden file test
/// fails. This output takes the form of differential images contained within a
/// `failures` directory that will be generated in the same location specified
/// by the golden key. The differential images include the master and test
/// images that were compared, as well as an isolated diff of detected pixels,
/// and a masked diff that overlays these detected pixels over the master image.
///
/// The following images are examples of a test failure output:
///
/// | File Name | Image Output |
/// |----------------------------|---------------|
/// | testName_masterImage.png | 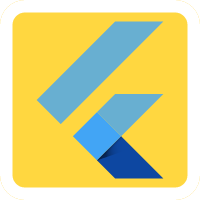 |
/// | testName_testImage.png | 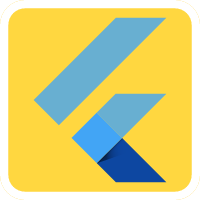 |
/// | testName_isolatedDiff.png | 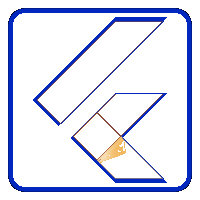 |
/// | testName_maskedDiff.png | 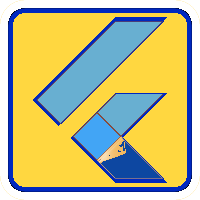 |
///
/// See also:
///
/// * [GoldenFileComparator], the abstract class that [LocalFileComparator]
/// implements.
/// * [matchesGoldenFile], the function from [flutter_test] that invokes the
/// comparator.
class
LocalFileComparator
extends
GoldenFileComparator
{
/// Creates a new [LocalFileComparator] for the specified [testFile].
///
...
...
packages/flutter_test/lib/src/matchers.dart
View file @
c8af729d
...
...
@@ -297,33 +297,60 @@ Matcher coversSameAreaAs(Path expectedPath, { @required Rect areaToCompare, int
///
/// For the case of a [Finder], the [Finder] must match exactly one widget and
/// the rendered image of the first [RepaintBoundary] ancestor of the widget is
/// treated as the image for the widget.
/// treated as the image for the widget. As such, you may choose to wrap a test
/// widget in a [RepaintBoundary] to specify a particular focus for the test.
///
/// [key] may be either a [Uri] or a [String] representation of a URI.
///
The
[key] may be either a [Uri] or a [String] representation of a URI.
///
///
[version] is a number that can be used to differentiate historical golden
///
files. This parameter is optional. Version numbers are used in golden file
///
tests for package:flutter. You can learn more about these tests [here]
/// (https://github.com/flutter/flutter/wiki/Writing-a-golden-file-test-for-package:flutter).
///
The [version] is a number that can be used to differentiate historical
///
golden files. This parameter is optional. Version numbers are used in golden
///
file tests for package:flutter. You can learn more about these tests
///
[here]
(https://github.com/flutter/flutter/wiki/Writing-a-golden-file-test-for-package:flutter).
///
/// This is an asynchronous matcher, meaning that callers should use
/// [expectLater] when using this matcher and await the future returned by
/// [expectLater].
///
/// ## Sample code
/// ## Golden File Testing
///
/// The term __golden file__ refers to a master image that is considered the true
/// rendering of a given widget, state, application, or other visual
/// representation you have chosen to capture.
///
/// The master golden image files that are tested against can be created or
/// updated by running `flutter test --update-goldens` on the test.
///
/// {@tool sample}
/// Sample invocations of [matchesGoldenFile].
///
/// ```dart
/// await expectLater(find.text('Save'), matchesGoldenFile('save.png'));
/// await expectLater(image, matchesGoldenFile('save.png'));
/// await expectLater(imageFuture, matchesGoldenFile('save.png'));
/// await expectLater(
/// find.text('Save'),
/// matchesGoldenFile('save.png'),
/// );
///
/// await expectLater(
/// image,
/// matchesGoldenFile('save.png'),
/// );
///
/// await expectLater(
/// imageFuture,
/// matchesGoldenFile('save.png'),
/// );
///
/// await expectLater(
/// find.byType(MyWidget),
/// matchesGoldenFile('goldens/myWidget.png'),
/// );
/// ```
///
/// Golden image files can be created or updated by running `flutter test
/// --update-goldens` on the test.
/// {@end-tool}
///
/// See also:
///
/// * [goldenFileComparator], which acts as the backend for this matcher.
/// * [GoldenFileComparator], which acts as the backend for this matcher.
/// * [LocalFileComparator], which is the default [GoldenFileComparator]
/// implementation for `flutter test`.
/// * [matchesReferenceImage], which should be used instead if you want to
/// verify that two different code paths create identical images.
/// * [flutter_test] for a discussion of test configurations, whereby callers
...
...
Write
Preview
Markdown
is supported
0%
Try again
or
attach a new file
Attach a file
Cancel
You are about to add
0
people
to the discussion. Proceed with caution.
Finish editing this message first!
Cancel
Please
register
or
sign in
to comment