Improve AndroidView resize jank. (#20284)
Resizing an AndroidView happens asynchronously (as it requires thread hopping from the ui thread to the platform thread). While waiting for the resize to complete the framework does exactly when the embedded view has been resized. This leads to pretty bad jank as the framework might paint the embedded view with a wrong size. We're working around this by freezing the texture frame while resizing until we are sure that the next frame has the new size. This is how it looks with the workaround: This is how it looks before and after the workaround: Before (janky) | After (less janky) :--------|---------: 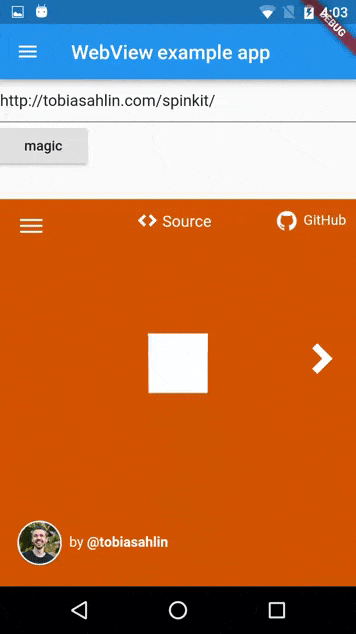 | 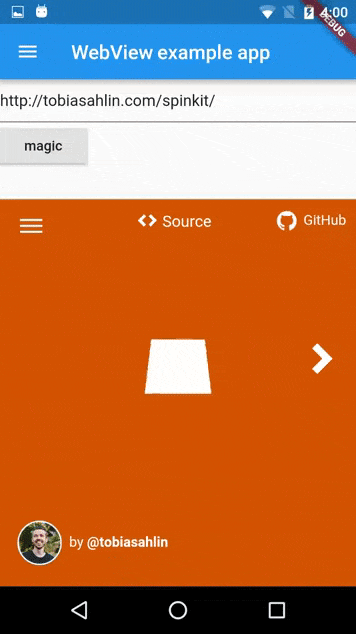 This depends on flutter/engine#5938. Additionaly right now the engine completes the resize call immediately, a following PR will change it to complete after a frame with the new size is ready.
Showing
Please register or sign in to comment