/// This example shows how to create a [Row] of [Icon]s in different colors and
/// This example shows how to create a [Row] of [Icon]s in different colors and
/// sizes. The first [Icon] uses a [semanticLabel] to announce in accessibility
/// sizes. The first [Icon] uses a [Icon.semanticLabel] to announce in accessibility
/// modes like TalkBack and VoiceOver.
/// modes like TalkBack and VoiceOver.
///
///
/// 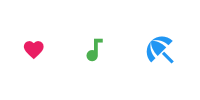
/// 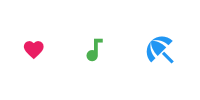