/// If [home], [routes], [onGenerateRoute], and [onUnknownRoute] are all null,
/// and [builder] is not null, then no [Navigator] is created.
///
/// {@tool sample}
/// This example shows how to create a [MaterialApp] that disables the "debug"
/// banner with a [home] route that will be displayed when the app is launched.
///
/// 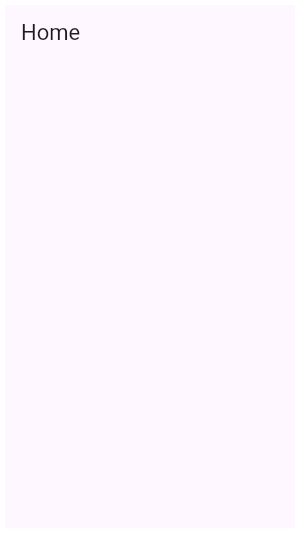
///
/// ```dart
/// MaterialApp(
/// home: Scaffold(
/// appBar: AppBar(
/// title: const Text('Home'),
/// ),
/// ),
/// debugShowCheckedModeBanner: false,
/// )
/// ```
/// {@end-tool}
///
/// {@tool sample}
/// This example shows how to create a [MaterialApp] that uses the [routes]
/// `Map` to define the "home" route and an "about" route.
///
/// ```dart
/// MaterialApp(
/// routes: <String, WidgetBuilder>{
/// '/': (BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(
/// title: const Text('Home Route'),
/// ),
/// );
/// },
/// '/about': (BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(
/// title: const Text('About Route'),
/// ),
/// );
/// }
/// },
/// )
/// ```
/// {@end-tool}
///
/// {@tool sample}
/// This example shows how to create a [MaterialApp] that defines a [theme] that
/// will be used for material widgets in the app.
///
/// 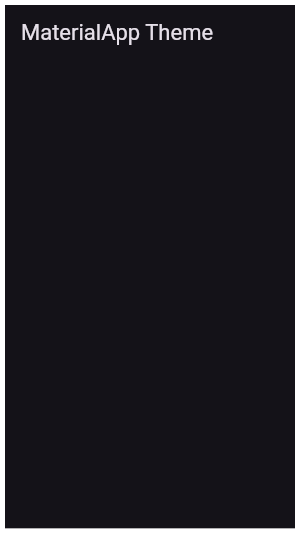
///
/// ```dart
/// MaterialApp(
/// theme: ThemeData(
/// brightness: Brightness.dark,
/// primaryColor: Colors.blueGrey
/// ),
/// home: Scaffold(
/// appBar: AppBar(
/// title: const Text('MaterialApp Theme'),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Scaffold], which provides standard app elements like an [AppBar] and a [Drawer].
/// This example shows how to make a simple [FloatingActionButton] in a
/// [Scaffold], with a pink [backgroundColor] and a thumbs up [Icon].
///
/// 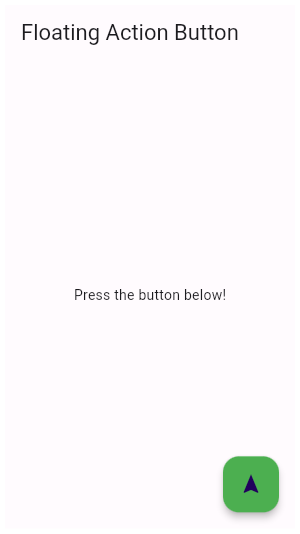
/// This example shows how to make an extended [FloatingActionButton] in a
/// [Scaffold], with a pink [backgroundColor] and a thumbs up [Icon] and a
/// [Scaffold], with a pink [backgroundColor], a thumbs up [Icon] and a
/// [Text] label.
///
/// 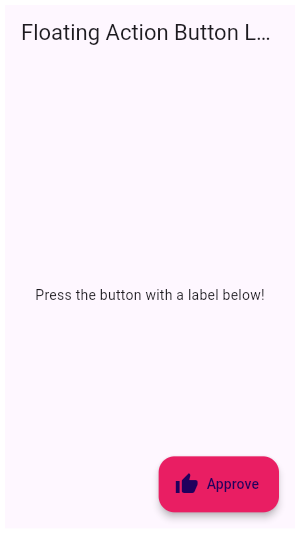
/// This example shows how to create a [Row] of [Icon]s in different colors and
/// sizes. The first [Icon] uses a [semanticLabel] to announce in accessibility
/// modes like TalkBack and VoiceOver.
///
/// 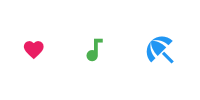
/// 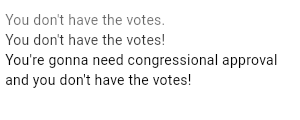
///
/// ```dart
/// RichText(
/// text: TextSpan(
...
...
@@ -96,6 +102,8 @@ const String _kColorBackgroundWarning = 'Cannot provide both a backgroundColor a
/// In this example, the ambient [DefaultTextStyle] is explicitly manipulated to
/// obtain a [TextStyle] that doubles the default font size.
///
/// 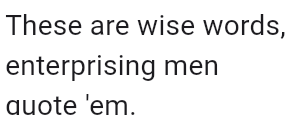
///
/// ```dart
/// Text(
/// 'These are wise words, enterprising men quote \'em.',
...
...
@@ -124,8 +132,8 @@ const String _kColorBackgroundWarning = 'Cannot provide both a backgroundColor a
///
/// ```dart
/// Text(
/// 'Don\'t act surprised, you guys, cuz I wrote \'em!',
/// style: TextStyle(fontSize: 10, height: 5.0),
/// 'Ladies and gentlemen, you coulda been anywhere in the world tonight, but you’re here with us in New York City.',
/// style: TextStyle(height: 5, fontSize: 10),
/// )
/// ```
/// {@end-tool}
...
...
@@ -145,6 +153,8 @@ const String _kColorBackgroundWarning = 'Cannot provide both a backgroundColor a
/// ambient [DefaultTextStyle], since no explicit style is given and [RichText]
/// does not automatically use the ambient [DefaultTextStyle].)
///
/// 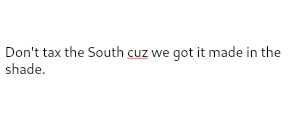
///
/// ```dart
/// RichText(
/// text: TextSpan(
...
...
@@ -266,6 +276,8 @@ const String _kColorBackgroundWarning = 'Cannot provide both a backgroundColor a
/// argument as shown in the example below:
///
/// {@tool sample}
/// 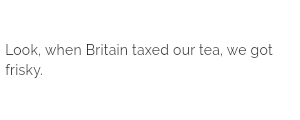
/// This example shows how to use [Icon] to create an addition icon, in the
/// color pink, and 30 x 30 pixels in size.
/// This example shows how to create a [Row] of [Icon]s in different colors and
/// sizes. The first [Icon] uses a [semanticLabel] to announce in accessibility
/// modes like TalkBack and VoiceOver.
///
/// 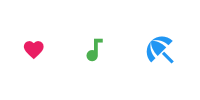
/// 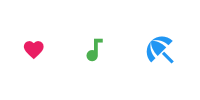