Added CupertinoDatepicker monthYear mode (flutter#93508) (#125603)
This PR adds a month and year mode to the CupertinoDatePicker. The monthYear mode is the date mode without the day of the month. 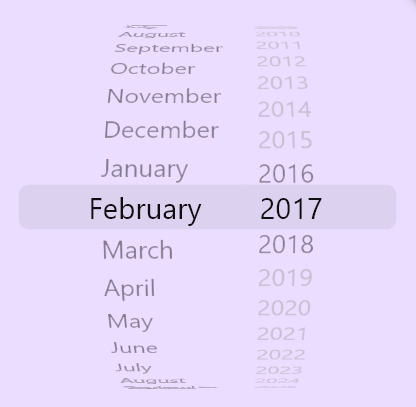 This feature was added at the request of: [Proposal] CupertinoDatePicker with month and year. #93508 One thing that I was unsure of was the use of the DatePickerDateOrder to determine the monthYear order. It could be considered a workaround since the DatePickerDateOrder is intended to order day, month, and year. This means that a developer could use the DatePickerDateOrder.dmy (day, month, year) or DatePickerDateOrder.mdy (month, day, year) to get the same result. At first I intended to add a DatePickerMonthYearOrder enum to the localizations, in addition to a new parameter for the CupertinoDatePicker for monthYearOrder, but I ended up reverting these changes (https://github.com/flutter/flutter/commit/1c61f1084e63fc4f6e394c3621a1ae626fd631a5) because I had not considered the effects of adding values to the localizations. I decided it may be better to not add an additional parameter (monthYearOrder) that would go mostly unused. I am very open to feedback or ideas on this matter.
Showing
This diff is collapsed.
Please register or sign in to comment