Shared state to support multi screen inspection (#129452)
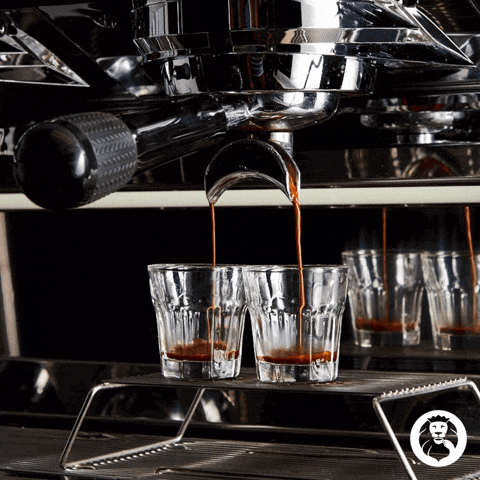 Fixes https://github.com/flutter/devtools/issues/5931 With Multi View applications on the way, we need to be able to manage the state of multiple Inspector widgets in a consistent way. Previously each Widget inspector would manage the state of it's own inspection. This made for a confusing and inconsistent experience when clicking on the widget inspector of different views. This PR changes the state management to the WidgetInspectorService static instance so that all widget inspectors can share that state. # Demo https://github.com/flutter/flutter/assets/1386322/70fd18dc-5827-4dcd-8cb7-ef20e6221291
Showing
Please register or sign in to comment