It's always ok to ask questions. Our systems are large, nobody will be
an expert in all the systems. Once you find the answer, document it in
the first place you looked. That way, the next person will be brought
up to speed even quicker.
[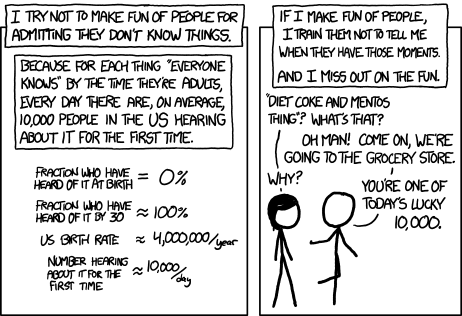](https://xkcd.com/1053/https://xkcd.com/1053/)
_See also: [Flutter's code of conduct](https://flutter.io/design-principles/#code-of-conduct)_
_See also: [Flutter's code of conduct](CODE_OF_CONDUCT.md)_
Welcome
Welcome
-------
-------
We gladly accept contributions via GitHub pull requests.
We invite you to join our team! Everyone is welcome to contribute code
via pull requests, to file issues on GitHub, to help people asking for
Please become familiar with our
help on our mailing lists or on Stack Overflow, to help triage,
[style guide](https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo) and
reproduce, or fix bugs that people have filed, to add to our
[design philosophy](https://flutter.io/design-principles/). These guidelines are intended to
documentation, or to help out in any other way.
keep the code consistent and avoid common pitfalls, and being familiar with them will
make everything much easier for you. If you have questions about our processes or are looking
We grant commit access (which includes full rights to the issue
for random tips and tricks, you may be interested in the [engine wiki](https://github.com/flutter/engine/wiki) and [framework wiki](https://github.com/flutter/flutter/wiki).
database, such as being able to edit labels) to people who have gained
our trust and demonstrated a commitment to Flutter.
This document will introduce you to the basic steps for developing for the Flutter framework (Dart).
If you're interested in developing for the Flutter engine (C++, Java, Objective C), please
This document focuses on what is needed to contribute by writing code
switch to [the engine repo's `CONTRIBUTING.md` document](https://github.com/flutter/engine/blob/master/CONTRIBUTING.md).
and submitting pull requests for the Flutter framework. For
information on contributing in other ways, see [the community page
If you have an itch, work on that. If you are just looking for something good to start with, consider
on flutter.io](https://flutter.io/community).
[the issues marked "easy fix"](https://github.com/flutter/flutter/issues?q=is%3Aopen+is%3Aissue+label%3A%22easy+fix%22+sort%3Areactions-%2B1-desc) in our issues list.
Developing for Flutter
Things you will need
----------------------
--------------------
To develop for Flutter, you will eventually need to become familiar
* Linux, Mac OS X, or Windows
with our processes and conventions. This section lists the documents
* git (used for source version control).
that describe these methodologies. The following list is ordered: you
* An IDE. We recommend [Android Studio with the Flutter plugin](https://flutter.io/using-ide/).
are strongly recommended to go through these documents in the order
* An ssh client (used to authenticate with GitHub).
presented.
* Python (used by some of our tools).
* The Android platform tools (see [Issue #55](https://github.com/flutter/flutter/issues/55)
1.[Our code of conduct](CODE_OF_CONDUCT.md), which stipulates explicitly
about downloading the Android platform tools automatically).
that everyone must be gracious, respectful, and professional. This
_If you're also working on the Flutter engine, you can use the
also documents our conflict resolution policy and encourages people
Flutter depends on. You can replicate what this script does by running
which includes advice for designing APIs for Flutter, and how to
`pub get` in each directory that contains a `pubspec.yaml` file.
format code in the framework.
* If you plan on using IntelliJ as your IDE, then also run
`flutter ide-config --overwrite` to create all of the IntelliJ configuration
In addition to the above, there are many pages on [our
files so you can open the main flutter directory as a project and run examples
Wiki](https://github.com/flutter/flutter/wiki/) that may be of
from within the IDE.
interest. For a curated list of pages see the sidebar on the wiki's
home page. They are more or less listed in order of importance.
Running the examples
--------------------
To run an example, switch to that example's directory, and use `flutter run`.
Make sure you have an emulator running, or a device connected over USB and
debugging enabled on that device.
*`cd examples/hello_world`
*`flutter run`
You can also specify a particular Dart file to run if you want to run an example
that doesn't have a `lib/main.dart` file using the `-t` command-line option. For
example, to run the `widgets/spinning_square.dart` example in the [examples/layers](examples/layers)
directory on a connected Android device, from that directory you would run:
`flutter run -t widgets/spinning_square.dart`
When running code from the examples directory, any changes you make to the
example code, as well as any changes to Dart code in the
[packages/flutter](packages/flutter) directory and subdirectories, will
automatically be picked when you relaunch the app. You can do the same for your
own code by mimicking the `pubspec.yaml` files in the `examples` subdirectories.
Running the analyzer
--------------------
When editing Flutter code, it's important to check the code with the
analyzer. There are two main ways to run it. In either case you will
want to run `flutter update-packages` first, or you will get bogus
error messages about core classes like Offset from `dart:ui`.
For a one-off, use `flutter analyze --flutter-repo`. This uses the `analysis_options.yaml` file
at the root of the repository for its configuration.
For continuous analysis, use `flutter analyze --flutter-repo --watch`. This uses normal
`analysis_options.yaml` files, and they can differ from package to package.
If you want to see how many members are missing dartdocs, you should use the first option,
providing the additional command `--dartdocs`.
If you omit the `--flutter-repo` option you may end up in a confusing state because that will
assume you want to check a single package and the flutter repository has several packages.
Running the tests
-----------------
To automatically find all files named `_test.dart` inside a package's `test/` subdirectory, and
run them inside the flutter shell as a test, use the `flutter test` command, e.g:
*`cd examples/stocks`
*`flutter test`
Individual tests can also be run directly, e.g. `flutter test lib/my_app_test.dart`
Flutter tests use [package:flutter_test](https://github.com/flutter/flutter/tree/master/packages/flutter_test)
which provides flutter-specific extensions on top of [package:test](https://pub.dartlang.org/packages/test).
`flutter test` runs tests inside the flutter shell. To debug tests in Observatory, use the `--start-paused`
option to start the test in a paused state and wait for connection from a debugger. This option lets you
set breakpoints before the test runs.
To run analysis and all the tests for the entire Flutter repository, the same way that Cirrus runs them, run `dart dev/bots/test.dart` and `dart dev/bots/analyze.dart`.
If you've built [your own flutter engine](#working-on-the-engine-and-the-framework-at-the-same-time), you
can pass `--local-engine` to change what flutter shell `flutter test` uses. For example,
if you built an engine in the `out/host_debug_unopt` directory, you can pass
`--local-engine=host_debug_unopt` to run the tests in that engine.
Flutter tests are headless, you won't see any UI. You can use
`print` to generate console output or you can interact with the DartVM
via observatory at [http://localhost:8181/](http://localhost:8181/).
Adding a test
-------------
To add a test to the Flutter package, create a file whose name
ends with `_test.dart` in the `packages/flutter/test` directory. The
test should have a `main` function and use the `flutter_test` package.
Working with flutter tools
--------------------------
The flutter tool itself is built when you run `flutter` for the first time and each time
you run `flutter upgrade`. If you want to alter and re-test the tool's behavior itself,
locally commit your tool changes in git and the tool will be rebuilt from Dart sources
in `packages/flutter_tools` the next time you run `flutter`.
Alternatively, delete the `bin/cache/flutter_tools.snapshot` file. Doing so will
force a rebuild of the tool from your local sources the next time you run `flutter`.
flutter_tools' tests run inside the Dart command line VM rather than in the
flutter shell. To run the tests, ensure that no devices are connected,
then navigate to `flutter_tools` and execute:
```shell
../../bin/cache/dart-sdk/bin/pub run test-j1
```
The pre-built flutter tool runs in release mode with the observatory off by default.
To enable debugging mode and the observatory on the `flutter` tool, uncomment the
`FLUTTER_TOOL_ARGS` line in the `bin/flutter` shell script.