Add ScrollController.onAttach & onDetach, samples/docs on listening/getting...
Add ScrollController.onAttach & onDetach, samples/docs on listening/getting scrolling info (#124823) This PR does a couple of things! https://user-images.githubusercontent.com/16964204/231897483-416287f9-50ce-468d-a714-2a4bc0f2e011.mov 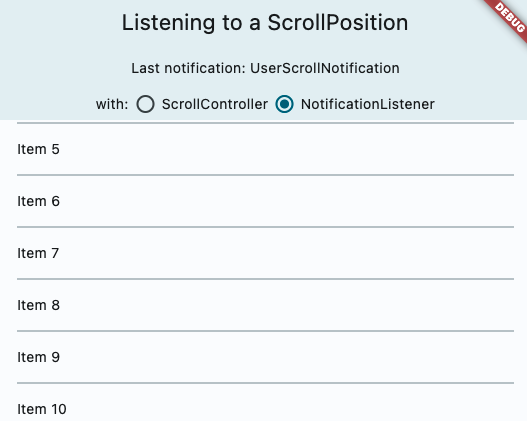 Fixes #20819 Fixes #41910 Fixes #121419 ### Adds ScrollController.onAttach and ScrollController.onDetach This resolves a long held pain point for developers. When using a scroll controller, there is not scroll position until the scrollable widget is built, and almost all methods of notification are only triggered when scrolling happens. Adding these two methods will help developers gain access to the scroll position when it is created. A common workaround for this was using a post frame callback to access controller.position after the first frame, but this is ripe for issues such as having multiple positions attached to the controller, or the scrollable no longer existing after that post frame callback. I think this can also be helpful for folks to debug cases when the scroll controller has multiple positions attached. In particular, this also resolves this commented case: https://github.com/flutter/flutter/issues/20819#issuecomment-417784218 The isScrollingNotifier is hard for developers to access. ### Docs & samples I was surprised we did not have samples on scroll notification or scroll controller, so I overhauled it and added a lot of docs on all the different ways to access scrolling information, when it is available and how they differ.
Showing
Please register or sign in to comment